编写一个c语言程序来判断字符串是否对称,要求同时使用栈和队列来实现
时间: 2024-11-15 20:33:35 浏览: 32
为了编写一个C语言程序来判断字符串是否对称,我们将使用两个数据结构:栈和队列。首先,我们需要了解这两个数据结构的基本操作:
1. 栈(Stack):后进先出(LIFO)的数据结构,常用于保存临时信息。
2. 队列(Queue):先进先出(FIFO)的数据结构,元素按添加顺序依次处理。
以下是使用栈和队列判断字符串对称的一个简单示例:
```c
#include <stdio.h>
#include <string.h>
#include <stdbool.h>
#include <stdlib.h>
// 使用队列结构
typedef struct Node {
char data;
struct Node *next;
} QueueNode;
struct Queue {
QueueNode *front;
QueueNode *rear;
};
void enqueue(Queue *queue, char c) {
QueueNode *new_node = (QueueNode*)malloc(sizeof(QueueNode));
new_node->data = c;
if (!queue->front) {
queue->front = queue->rear = new_node;
} else {
queue->rear->next = new_node;
queue->rear = new_node;
}
}
char dequeue(Queue *queue) {
if (queue->front == NULL) {
return '\0';
}
QueueNode *temp = queue->front;
queue->front = queue->front->next;
if (queue->front == NULL) {
queue->rear = NULL;
}
free(temp);
return temp->data;
}
bool isPalindrome(char str[]) {
// 使用栈存储字符
int stack_size = 0;
StackNode *stack = NULL; // 假设有一个名为StackNode的类似队列节点的结构
// 使用队列辅助
Queue queue;
// 初始化队列头和尾
queue.front = queue.rear = NULL;
for (int i = 0; str[i] != '\0'; ++i) {
// 入栈
push(&stack, str[i]);
stack_size++;
// 出队并比较字符
while (stack_size > 0 && dequeue(&queue) != '\0') {
if (dequeue(&queue) != str[i]) {
return false;
}
stack_size--;
}
}
// 如果队列为空,说明所有字符都已检查,字符串是对称的
return stack_size == 0;
}
int main() {
char input[100];
printf("Enter a string: ");
fgets(input, sizeof(input), stdin);
input[strcspn(input, "\n")] = '\0'; // 移除fgets获取的换行符
if (isPalindrome(input)) {
printf("%s is a palindrome.\n", input);
} else {
printf("%s is not a palindrome.\n", input);
}
return 0;
}
```
阅读全文
相关推荐
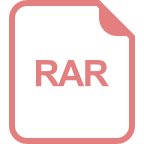
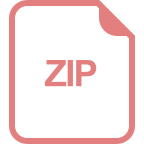
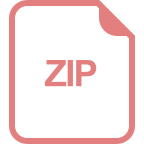















