用c语言编写一个算法利用顺序栈判断一个字符串是否对称串
时间: 2024-02-22 21:55:14 浏览: 51
好的,我可以为您提供这样一个算法:
1. 定义一个顺序栈,用来存储字符串中的字符。
2. 从字符串的左边开始,将每个字符依次入栈。
3. 从字符串的右边开始,依次取出每个字符,与栈顶元素进行比较。
4. 如果相同,则继续比较下一个字符,直到字符串全部比较完毕。
5. 如果不同,则表示该字符串不是对称串,直接返回结果。
6. 如果最后栈为空,表示该字符串是对称串,返回结果为是;否则返回结果为否。
下面是用C语言实现的代码:
```c
#include <stdio.h>
#include <string.h>
#define MAXSIZE 100
typedef struct {
char data[MAXSIZE];
int top;
} SqStack;
void InitStack(SqStack *s) {
s->top = -1;
}
void Push(SqStack *s, char e) {
if (s->top == MAXSIZE - 1) {
printf("Stack is full.\n");
return;
}
s->data[++s->top] = e;
}
char Pop(SqStack *s) {
if (s->top == -1) {
printf("Stack is empty.\n");
return '\0';
}
return s->data[s->top--];
}
int StackEmpty(SqStack *s) {
if (s->top == -1)
return 1;
else
return 0;
}
int Symmetric(char *str) {
SqStack s;
InitStack(&s);
int len = strlen(str);
int i;
for (i = 0; i < len/2; i++) {
Push(&s, str[i]);
}
if (len % 2 != 0) {
i++;
}
while (i < len) {
char temp = Pop(&s);
if (temp != str[i]) {
return 0;
}
i++;
}
if (StackEmpty(&s)) {
return 1;
} else {
return 0;
}
}
int main() {
char str[MAXSIZE];
printf("Please input a string: ");
scanf("%s", str);
if (Symmetric(str)) {
printf("The string is symmetric.\n");
} else {
printf("The string is not symmetric.\n");
}
return 0;
}
```
您可以尝试输入一些字符串进行测试,如:123321、abccba、12321等。
相关推荐
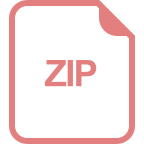
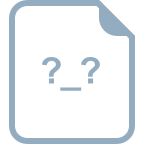
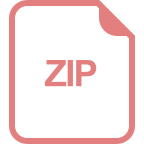














