用C++实现一个加密算法
时间: 2023-03-22 19:02:36 浏览: 117
C语言中常用的加密算法有AES、DES、RSA等。
其中AES(Advanced Encryption Standard)是一种高级加密标准,采用对称密钥分组密码体制,常用于对数据进行加密和解密。
下面是一个简单的AES加密示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/aes.h>
int main(int argc, char const *argv[]) {
// 明文
unsigned char *plainText = "hello world!";
// 密钥
unsigned char *key = "0123456789abcdef";
// 密文
unsigned char cipherText[128];
// 解密后的明文
unsigned char decryptedText[128];
// 初始化AES结构体
AES_KEY aes;
AES_set_encrypt_key(key, 128, &aes);
// 加密
AES_encrypt(plainText, cipherText, &aes);
printf("Ciphertext: %s\n", cipherText);
// 设置解密密钥
AES_set_decrypt_key(key, 128, &aes);
// 解密
AES_decrypt(cipherText, decryptedText, &aes);
printf("Decrypted Text: %s\n", decryptedText);
return 0;
}
```
上面程序实现了对"hello world!"字符串的加密与解密,密钥是"0123456789abcdef"
这仅仅是一个非常简单的例子,并不能用于实际应用。
真实使用时请加上相关的判断和错误处理。
相关推荐
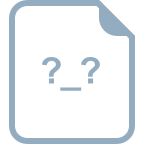
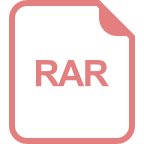
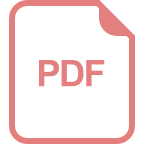














