vue3点击组件返回数据
时间: 2024-12-13 10:25:54 浏览: 4
在Vue 3中,当你需要在组件之间传递数据并响应用户的点击操作时,可以使用props、事件总线(Event Bus)或者自定义的Vuex store来进行通信。以下是几种常见的方式:
1. **Props** (属性): 如果父子组件间的数据流动单向,你可以从父组件将数据作为props传给子组件。当用户点击子组件时,可以在子组件内部处理数据,并通过触发一个emit事件通知父组件更新。
```javascript
// 父组件
<ChildComponent :data="parentData" @childClick="handleChildClick" />
// 子组件
<template>
<button @click="doSomething">点击我</button>
</template>
<script>
export default {
props: ['data'],
methods: {
doSomething() {
// 处理数据...
this.$emit('childClick', newData);
},
},
// 当然,你需要在父组件中处理`childClick`事件
}
</script>
```
2. **Event Bus**: 如果需要跨级组件间的通讯,可以使用事件总线。例如,你可以创建一个独立的Vue实例用于发布和订阅事件。
```javascript
// 创建一个全局Event Bus
import { createApp } from 'vue';
const eventBus = new Vue();
// 使用
eventBus.$on('parentAction', data => {
// 父组件处理点击事件
});
// 触发事件
methods: {
handleClick() {
eventBus.$emit('parentAction', someData);
}
}
```
3. **Vuex**: 对于更复杂的应用状态管理,可以考虑使用Vuex。在Vuex store中保存状态,然后组件通过actions和mutations来读取和修改状态。
```javascript
// Vuex Store
state: {
userData: null,
},
actions: {
fetchData({ commit }, payload) {
axios.get('/api/data')
.then(response => commit('setData', response.data));
},
},
methods: {
onClick() {
this.$store.dispatch('fetchData');
}
}
// 组件中
created() {
this.getUserData();
},
computed: {
userData() {
return this.$store.state.userData;
},
}
```
阅读全文
相关推荐
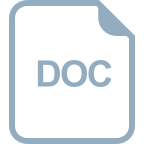
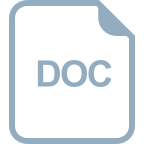
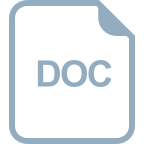
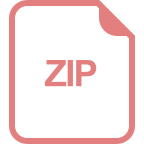
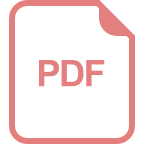
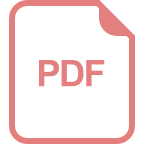
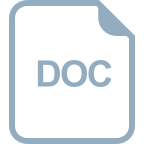
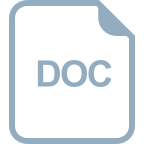
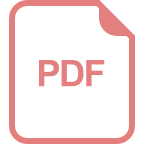
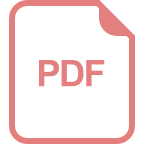
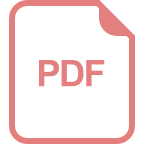
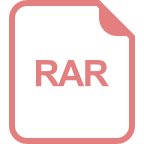
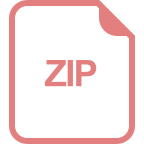
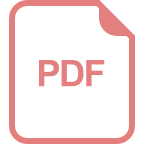
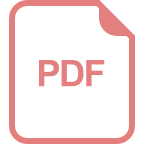
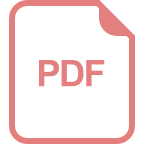
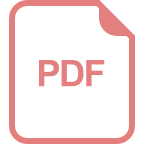
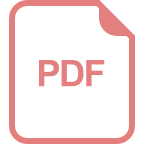
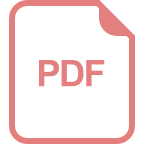