python 按钮 鼠标悬停
时间: 2024-06-27 15:01:29 浏览: 13
在Python中,通常我们不会直接创建像Windows按钮或鼠标悬停那样的用户界面元素,因为这通常是图形用户界面(GUI)库如Tkinter、PyQt、wxPython等的责任。然而,如果你是在使用这些库构建GUI应用,你可以实现类似的效果。
例如,在Tkinter中,你可以使用`Button`和`bind()`方法来模拟鼠标悬停事件:
```python
import tkinter as tk
def on_hover(event):
# 这里是处理鼠标悬停的代码,比如更新文本或颜色
button.config(text="Hovering...")
root = tk.Tk()
button = tk.Button(root, text="Click me")
button.pack()
# 绑定鼠标进入和离开事件
button.bind('<Enter>', on_hover)
button.bind('<Leave>', lambda event: button.config(text="Click me")) # 重置为默认状态
root.mainloop()
```
在这个例子中,当鼠标指针移到按钮上时,`on_hover`函数会被调用,你可以根据需要更新按钮的文字或其他属性。离开按钮时,会恢复到原来的文本(在这个例子中是"Click me")。
相关问题
pyqt5 鼠标悬停按钮变色
以下是一个pyqt5鼠标悬停按钮变色的例子:
```python
from PyQt5.QtWidgets import QApplication, QPushButton, QWidget
from PyQt5.QtGui import QPalette, QColor
class HoverButton(QPushButton):
def __init__(self, parent=None):
super(HoverButton, self).__init__(parent)
self.setMouseTracking(True)
def enterEvent(self, event):
self.setStyleSheet("background-color: yellow;")
def leaveEvent(self, event):
self.setStyleSheet("background-color: none;")
if __name__ == '__main__':
app = QApplication([])
widget = QWidget()
layout = QVBoxLayout(widget)
button = HoverButton(widget)
button.setText("Hover over me!")
layout.addWidget(button)
widget.show()
app.exec_()
```
当鼠标悬停在按钮上时,按钮的背景颜色将变为黄色。当鼠标离开按钮时,按钮的背景颜色将恢复为默认值。
PyQt5 QPushButton设置鼠标悬停时按钮文字改变颜色
可以使用QPushButton的setStyleSheet()方法来设置鼠标悬停时按钮文字的颜色。具体的实现方法如下:
```python
from PyQt5.QtWidgets import QApplication, QPushButton
from PyQt5.QtGui import QPalette, QColor
app = QApplication([])
button = QPushButton('Button')
button.setStyleSheet('''
QPushButton {
color: black;
}
QPushButton:hover {
color: white;
}
''')
button.show()
app.exec_()
```
在上面的代码中,我们使用setStyleSheet()方法设置了两个样式表。第一个样式表设置了按钮的默认文字颜色为黑色。第二个样式表中的:hover伪类设置了鼠标悬停时按钮的文字颜色为白色。
你可以根据自己的需要调整这些样式表来实现不同的效果。
相关推荐
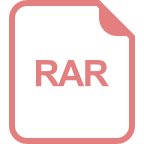
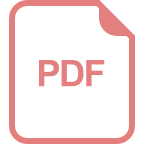












