pygame 开始游戏按钮
时间: 2023-08-14 19:08:23 浏览: 134
要在 Pygame 中创建一个开始游戏按钮,可以按照以下步骤进行:
1. 创建一个按钮图片,可以使用任何图像编辑器(如 Photoshop 或 GIMP)。按钮图片应该包含常规状态(未选中)和悬停状态(鼠标悬停在按钮上)的图像。
2. 在 Pygame 中加载按钮图像,并将其绘制到屏幕上。您可以使用 Pygame 的 `pygame.image.load()` 函数来加载图像,然后使用 `pygame.Surface.blit()` 函数将其绘制到屏幕上。
3. 创建一个 Pygame 矩形对象,该对象与按钮图像的位置和大小相匹配。您可以使用 `pygame.Rect()` 函数创建矩形对象,并指定其左上角的位置和宽度/高度。
4. 在主循环中,检查鼠标位置是否在按钮矩形内。如果是,则将按钮图像切换到悬停状态,并在单击按钮时执行游戏开始操作。
这是一个简单的示例代码,可以帮助您开始:
```python
import pygame
# 初始化 Pygame
pygame.init()
# 设置屏幕大小
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
# 加载按钮图像
button_image = pygame.image.load('button.png')
button_rect = button_image.get_rect()
# 设置按钮位置
button_rect.centerx = screen_width // 2
button_rect.centery = screen_height // 2
# 主循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.MOUSEBUTTONDOWN:
# 如果鼠标单击了按钮,执行游戏开始操作
if button_rect.collidepoint(event.pos):
start_game()
# 绘制屏幕
screen.fill((255, 255, 255))
screen.blit(button_image, button_rect)
# 更新屏幕
pygame.display.update()
```
请注意,此示例代码中的 `start_game()` 函数是您的游戏开始操作的占位符。您需要将其替换为实际的游戏逻辑。
阅读全文
相关推荐
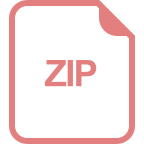
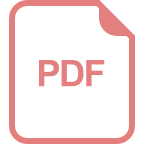
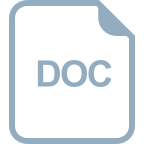












