Java字符数组加密
时间: 2024-09-25 15:03:12 浏览: 35
在Java中,我们可以使用多种技术对字符数组进行加密,常见的有基于密钥的算法如AES(高级加密标准),或者简单的替换、移位等替换密码法。以下是一个简单的例子,使用凯撒密码(Caesar Cipher)作为加密方法:
```java
public class CaesarCipher {
private int shift;
public CaesarCipher(int shift) {
this.shift = shift;
}
// 加密函数
public char[] encrypt(char[] plaintext) {
char[] ciphertext = new char[plaintext.length];
for (int i = 0; i < plaintext.length; i++) {
if (Character.isLetter(plaintext[i])) { // 只处理字母
char shiftedChar = (plaintext[i] + shift - 'a') % 26 + 'a'; // 字母表循环移位
ciphertext[i] = shiftedChar;
} else {
ciphertext[i] = plaintext[i]; // 非字母字符保持不变
}
}
return ciphertext;
}
// 解密函数类似,只需将shift取反
public char[] decrypt(char[] ciphertext) {
return encrypt(ciphertext, -shift);
}
private char[] encrypt(char[] data, int shift) {
// ... 实现同样的逻辑,只是shift方向相反
}
}
```
在这个例子中,`encrypt()`方法接受一个字符数组并按照指定的位移(shift)对其进行加密。用户可以根据需要设置不同的位移值以增加加密强度。
阅读全文
相关推荐
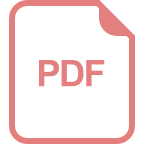
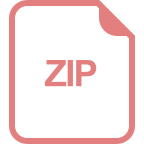
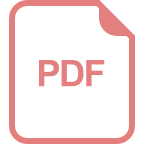

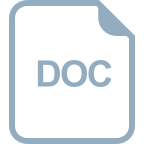
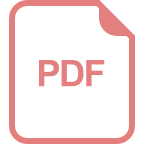
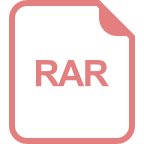
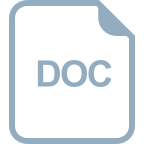
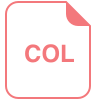
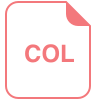
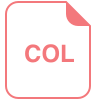
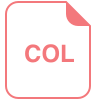
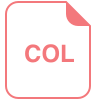
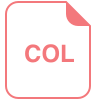


