字符数组最佳实践总结:提升代码质量和可维护性
发布时间: 2024-07-13 01:18:42 阅读量: 28 订阅数: 31 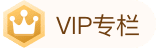
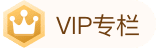

# 1. 字符数组基础**
字符数组是计算机中用于存储字符序列的数据结构。它们由连续的内存块组成,每个块存储一个字符。字符数组是字符串处理和文本操作中的基本数据类型。
字符数组的优势在于它们可以高效地存储和操作大量字符数据。与字符串(不可变的字符序列)不同,字符数组是可变的,允许修改和重新分配。这使得它们在需要动态处理字符串或需要高效内存管理的情况下非常有用。
字符数组的常见用途包括:
- 存储用户输入和输出
- 处理文本文件和数据
- 创建和操作字符串
- 实现自定义数据结构,如链表和栈
# 2. 字符数组的常见问题和解决方案
字符数组在使用过程中可能会遇到一些常见问题,如果不及时解决,可能会导致代码质量下降和维护困难。本章将介绍两种常见的字符数组问题:缓冲区溢出和内存泄漏,并提供相应的解决方案。
### 2.1 缓冲区溢出
**2.1.1 缓冲区溢出的原因和后果**
缓冲区溢出是指程序试图将数据写入超出其分配内存范围的缓冲区。这通常发生在程序没有正确检查用户输入或其他外部数据的长度时。当缓冲区被溢出时,它可能会覆盖相邻的内存区域,导致程序崩溃、数据损坏或安全漏洞。
**2.1.2 防止缓冲区溢出的措施**
防止缓冲区溢出的主要措施是:
- **检查输入长度:**在写入缓冲区之前,始终检查用户输入或其他外部数据的长度,确保其不超过缓冲区的容量。
- **使用安全函数:**使用具有边界检查功能的安全函数,例如 `strncpy()` 和 `strncat()`,这些函数可以防止写入超出缓冲区范围的数据。
- **动态分配缓冲区:**如果无法确定输入数据的长度,可以使用动态内存分配来分配一个足够大的缓冲区,以避免缓冲区溢出。
- **使用编译器标志:**一些编译器提供编译器标志,例如 `-fstack-protector`,可以帮助检测和防止缓冲区溢出。
### 2.2 内存泄漏
**2.2.1 内存泄漏的类型和原因**
内存泄漏是指程序分配了内存但没有释放,导致内存被浪费。内存泄漏可能发生在以下情况下:
- **未释放动态分配的内存:**当程序使用 `malloc()` 或 `new` 等函数分配内存时,必须在使用完成后使用 `free()` 或 `delete` 释放该内存。如果不释放,就会发生内存泄漏。
- **循环引用:**当两个或多个对象相互引用时,可能会创建循环引用,导致任何对象都无法被释放,从而发生内存泄漏。
- **全局变量:**全局变量在程序的整个生命周期中都存在,如果不再需要,但没有释放,也会导致内存泄漏。
**2.2.2 检测和修复内存泄漏的方法**
检测和修复内存泄漏的方法包括:
- **使用内存调试工具:**使用内存调试工具,例如 Valgrind 或 AddressSanitizer,可以帮助检测和定位内存泄漏。
- **跟踪内存分配和释放:**使用工具或手动跟踪内存分配和释放,可以帮助识别未释放的内存。
- **使用智能指针:**使用智能指针,例如 `std::unique_ptr` 和 `std::shared_ptr`,可以自动管理内存释放,避免内存泄漏。
- **定期释放内存:**在程序的适当位置定期释放不再需要的内存,可以防止内存泄漏。
# 3. 字符数组的优化技术
### 3.1 字符数组的内存管理
#### 3.1.1 动态内存分配和释放
在某些情况下,字符数组的长度可能无法提前确定,或者需要根据运行时的情况进行动态调整。此时,可以使用动态内存分配和释放机制来管理字符数组的内存。
```cpp
char *str = (char *)malloc(sizeof(char) * 100); // 分配 100 个字符的内存
if (str == NULL) {
// 内存分配失败,处理错误
}
// 使用字符数组
free(str); // 释放分配的内存
```
**参数说明:**
* `malloc`:动态内存分配函数,返回指向分配内存块的指针。
* `sizeof(char)`:单个字符的大小,通常为 1 字节。
* `100`:分配的字符数组长度。
**逻辑分析:**
1. `malloc` 函数分配指定大小的内存块,并返回指向该内存块的指针。
2. 如果内存分配成功,将指针存储在 `str` 变量中。
3. 如果内存分配失败(`malloc` 返回 `NULL`),则处理错误情况。
4. 使用 `str` 指针访问和操作字符数组。
5. 使用 `free` 函数释放分配的内存,以避免内存泄漏。
#### 3.1.2 引用计数和垃圾回收
在某些编程语言中,例如 Python 和 Java,使用引用计数和垃圾回收机制来管理字符数组的内存。
* **引用计数:**每个字符数组都有一个引用计数,表示引用该数组的变量数量。当引用计数为 0 时,表明该数组不再被使用,可以被垃圾回收器回收。
* **垃圾回收:**垃圾回收器定期扫描内存,识别并回收不再被引用的对象,包括字符数组。
**优点:**
* 自动内存管理,无需手动释放内存。
0
0
相关推荐
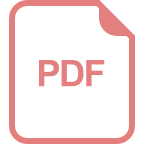
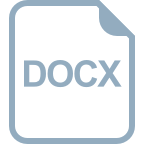
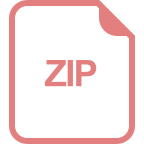
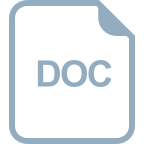
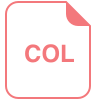
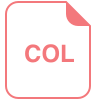
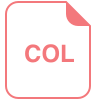
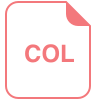
