@TableField(exist = "false") private String deptname;false报错解决方法
时间: 2023-12-14 13:30:40 浏览: 33
这个报错是因为在实体类中,@TableField注解的exist属性默认为true,表示该字段在数据库中存在,但是你的实体类中的该字段在数据库中不存在,所以需要将exist属性设置为false。修改后的代码如下:
@TableField(exist = false)
private String deptname;
相关问题
@TableField(exist = false
@TableField(exist = false)注解是用来解决表中表的问题的。它可以在加载bean属性时使用,表示当前属性不是数据库的字段,但在项目中必须使用。这样可以将一个数据表当作一个字段来输出,实现表中表数据的输出。当在新增等使用bean的时候,mybatis-plus会忽略这个注解,不会报错。
举个例子,比如在一个实体类中,使用了@TableField(exist = false)注解来定义一些List类型的属性,如:
@TableField(exist = false) private List<CtnOrderBoxInfo> boxInfos;
@TableField(exist = false) private List<CtnOrderFee> fees;
@TableField(exist = false) private List<CtnOrderAttachInfo> orderAttachInfos;
@TableField(exist = false) private List<CtnRemarksHistory> remarksHistories;
这样就可以将boxInfos、fees、orderAttachInfos和remarksHistories当作一个字段来输出,而不是作为数据库的字段。
@TableField(exist = false)注解含义
@TableField(exist = false)注解的含义是告诉MyBatis-Plus该字段在数据库表中不存在,即该字段不会被自动映射到数据库表中。这个注解通常用于实体类中的一些非数据库字段,例如一些计算字段或者临时字段。这样做可以避免在数据库中创建无用的字段,同时也可以提高查询效率。
下面是一个示例代码,演示了如何在实体类中使用@TableField(exist = false)注解:
```java
import com.baomidou.mybatisplus.annotation.TableField;
public class User {
private Long id; private String username;
@TableField(exist = false)
private String virtualField;
// 省略Getter和Setter等其他方法
}
```
在这个示例中,`virtualField`字段被标记为不存在于数据库表中,因此它不会被自动映射到数据库表中。
相关推荐
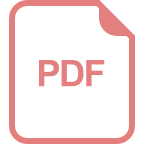
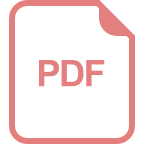
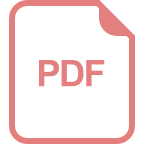












