c++的string的replace
时间: 2024-12-08 13:25:10 浏览: 15
在C++标准库中,`std::string` 类有一个名为 `replace()` 的成员函数,用于替换字符串中的一部分内容。其基本语法如下:
```cpp
size_t pos = start_index;
size_t n = count_of_characters_to_replace;
std::string str;
str.replace(pos, n, replacement_string);
```
- `pos`:表示要开始替换的起始索引(从0开始计数)。
- `n`:指定了要替换的字符数。如果为0,则不会进行替换。
- `replacement_string`:是要替换成的新字符串。
举个例子:
```cpp
std::string original_str = "Hello, World!";
std::string replacement = "Universe";
original_str.replace(7, 5, replacement); // 从第8个字符(包括)开始替换,共5个字符
```
这将把原字符串中的 "World!" 替换为 "Universe",最终结果为 "Hello, Universe!"。
`replace()` 函数并不会改变原始字符串的长度,而是覆盖指定范围内的原有字符,并在替换后的字符串结尾处追加剩余的原始字符串部分。
相关问题
C++string replace
你好,C知道!关于C语言中的字符串替换,你可以使用库函数`str_replace`来实现。这个函数不是C标准库的一部分,但你可以自己实现它。以下是一个示例代码:
```c
#include <stdio.h>
#include <string.h>
void str_replace(char *str, const char *old, const char *new) {
char result[100];
int i, count = 0;
int new_len = strlen(new);
int old_len = strlen(old);
for (i = 0; str[i] != '\0'; i++) {
if (strstr(&str[i], old) == &str[i]) {
count++;
i += old_len - 1;
}
}
char *temp = result;
while (*str) {
if (strstr(str, old) == str) {
strcpy(temp, new);
temp += new_len;
str += old_len;
} else {
*temp++ = *str++;
}
}
*temp = '\0';
strcpy(str, result);
}
int main() {
char str[] = "Hello World";
str_replace(str, "World", "Universe");
printf("%s\n", str);
return 0;
}
```
这段代码演示了如何使用自定义的`str_replace`函数来将字符串中的旧子串替换为新子串。在这个例子中,我们将“World”替换为“Universe”,输出结果将会是“Hello Universe”。
希望这个例子能对你有所帮助!如果还有其他问题,请随时提问。
c++ string replace
C++ 中的 std::string 类有一个成员函数 replace(),可以用来替换字符串中的一些字符。
语法:
string.replace(position, length, string);
string.replace(iterator first, iterator last, string);
参数说明:
- position: 要替换的字符串的起始位置。
- length: 要替换的字符串的长度。
- string: 用来替换的字符串。
- iterator first: 要替换的字符串的起始位置(迭代器)
- iterator last: 要替换的字符串的终止位置(迭代器)
例子:
```
#include <iostream>
#include <string>
int main()
{
std::string str = "C++ is a programming language";
std::string toReplace = "programming";
std::string replaceWith = "powerful";
int pos = str.find(toReplace);
if (pos != std::string::npos)
{
str.replace(pos, toReplace.length(), replaceWith);
}
std::cout << str << std::endl;
return 0;
}
```
运行结果:
```
C++ is a powerful language
```
结论:
replace()函数可以在C++中的string类中替换一些字符。
阅读全文
相关推荐






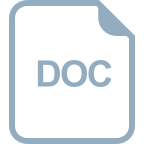









