c++ string replace_all
时间: 2023-11-26 21:02:27 浏览: 72
To replace all occurrences of a substring in a C string with a new substring, you can use the following code:
```c
#include <stdio.h>
#include <string.h>
void replace_all(char *str, const char *old_sub, const char *new_sub) {
char *pos = str;
int old_len = strlen(old_sub);
int new_len = strlen(new_sub);
while ((pos = strstr(pos, old_sub)) != NULL) {
memmove(pos + new_len, pos + old_len, strlen(pos + old_len) + 1);
memcpy(pos, new_sub, new_len);
pos += new_len;
}
}
int main() {
char str[] = "Hello, world! Hello, world! Hello, world!";
replace_all(str, "Hello", "Hi");
printf("%s\n", str); // Output: "Hi, world! Hi, world! Hi, world!"
return 0;
}
```
The `replace_all()` function takes a C string `str` and two other C strings `old_sub` and `new_sub`. It searches for all occurrences of `old_sub` in `str` using `strstr()` and replaces them with `new_sub` using `memmove()` and `memcpy()`. The function modifies `str` in place.
阅读全文
相关推荐
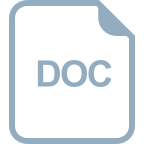
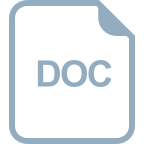
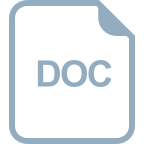

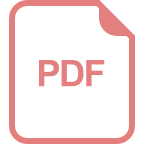

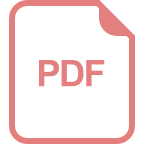
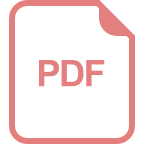
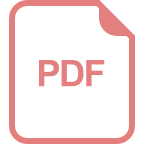
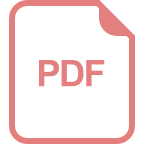
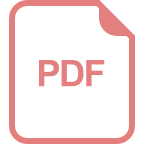








