用c++解决下述问题:描述 Given a string S, print the results of the operations. Here are the operations: Insert x i: Insert the character x to the position i; Erase x: Delete all characters x in S; Replace x y: Replace all characters x by y S; Size: Print the size of S; Reverse: Print the reverse of S; Don't change S in this operation; Sub i j: Print the substring of S from position i to j; Find str: Print the starting postion of the "first" str in S; If str is not a substring of S, print -1. 输入 The first line of each test case contains a string S. Then 5 lines follow. The first line includes a character x and an integer i, meaning "Insert x i"; The second line is a character x, meaning "Erase x"; The third line are two characters x and y, meaning "Replace x y"; The fourth line are two integers i and j, meaning "Sub i j"; The last line is a string str, meaning "Find str". All the input characters are English uppercase or lowercase letters. The length of the string will not exceed 100. Inputs will be terminated at the end of the file. 输出 For each test case, you must print "Case i:" in the first line. Then for each operation, print its result in one line. There will be a blank line after each test case.
时间: 2024-03-11 13:51:21 浏览: 120
很高兴为您解答这道题目,以下是C++代码实现:
```
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
int main() {
string S;
int case_num = 1;
while (getline(cin, S)) {
cout << "Case " << case_num << ":" << endl;
case_num++;
string x, y, str;
int i, j;
// Insert x i
cin >> x >> i;
S.insert(i, x);
cout << S << endl;
// Erase x
cin >> x;
S.erase(remove(S.begin(), S.end(), x[0]), S.end());
cout << S << endl;
// Replace x y
cin >> x >> y;
replace(S.begin(), S.end(), x[0], y[0]);
cout << S << endl;
// Size
cout << S.size() << endl;
// Reverse
reverse(S.begin(), S.end());
cout << S << endl;
reverse(S.begin(), S.end());
// Sub i j
cin >> i >> j;
cout << S.substr(i, j-i+1) << endl;
// Find str
cin >> str;
int pos = S.find(str);
if (pos == string::npos) {
cout << -1 << endl;
} else {
cout << pos << endl;
}
// Blank line
cout << endl;
cin.ignore();
}
return 0;
}
```
该代码使用了字符串的 STL 库,包括 `insert`,`erase`,`replace`,`size`,`reverse`,`substr` 和 `find` 函数。其中,`erase` 函数使用了 `remove` 函数来删除所有字符 x。
程序的输入方式是使用 `getline` 函数读取一行字符串,然后使用 `cin` 读取操作所需的参数。最后,使用 `cout` 输出结果,并在每个测试用例之间输出一个空行。
希望能够帮到您!
阅读全文
相关推荐
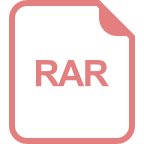
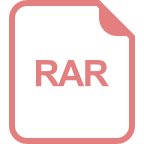
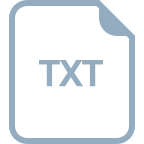
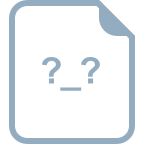
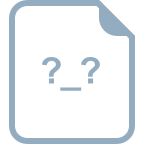
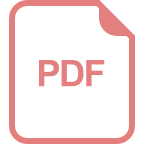
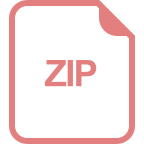
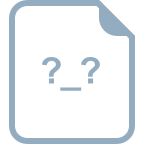
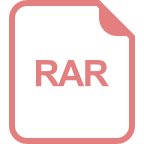
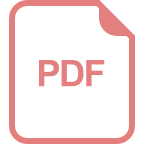
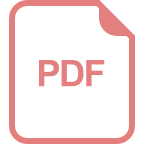
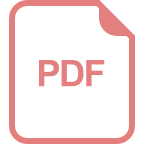
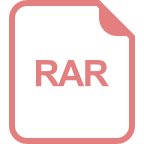
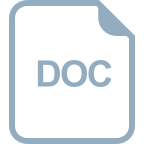