请你根据我给你的KS106的说明书,写stm32f103与KS106进行RS485通讯的驱动程序,包括.c和.h以及main,你需要给我这三个程序,实现stm32接收KS106读到的数据
时间: 2024-11-15 07:31:01 浏览: 30
好的,以下是基于STM32F103与KS106进行RS485通讯的完整驱动程序,包括`.h`文件、`.c`文件和`main`函数。这些代码实现了STM32接收KS106读到的数据的功能。
### `ks106_rs485.h`
```c
#ifndef __KS106_RS485_H__
#define __KS106_RS485_H__
#include "stm32f10x.h"
#define KS106_ADDRESS 0xE8
#define RS485_BAUD_RATE 9600
void KS106_RS485_Init(void);
uint16_t KS106_ReadDistance(void);
#endif /* __KS106_RS485_H__ */
```
### `ks106_rs485.c`
```c
#include "ks106_rs485.h"
#include "usart.h"
#include "delay.h"
// UART1 initialization for RS485 communication
void USART1_RS485_Init(uint32_t baudrate) {
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
// Enable GPIOA and USART1 clocks
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_USART1, ENABLE);
// Configure USART1 Tx (PA9) as alternate function push-pull
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// Configure USART1 Rx (PA10) as input floating
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// Configure USART1
USART_InitStructure.USART_BaudRate = baudrate;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
// Enable USART1
USART_Cmd(USART1, ENABLE);
}
// Send a byte over RS485
void RS485_SendByte(uint8_t data) {
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
USART_SendData(USART1, data);
}
// Read a byte from RS485
uint8_t RS485_ReadByte(void) {
while (USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET);
return USART_ReceiveData(USART1);
}
// Initialize RS485 communication with KS106
void KS106_RS485_Init(void) {
USART1_RS485_Init(RS485_BAUD_RATE);
}
// Read distance from KS106
uint16_t KS106_ReadDistance(void) {
uint8_t high_byte, low_byte;
uint16_t distance;
// Send command to read distance
RS485_SendByte(KS106_ADDRESS << 1); // Write address
RS485_SendByte(0x02); // Register 2
RS485_SendByte(0x30); // Command 0x30
// Wait for response
delay_ms(1);
// Read high byte
RS485_SendByte((KS106_ADDRESS << 1) | 1); // Read address
high_byte = RS485_ReadByte();
// Read low byte
low_byte = RS485_ReadByte();
// Combine bytes to form distance
distance = (high_byte << 8) | low_byte;
return distance;
}
```
### `main.c`
```c
#include "stm32f10x.h"
#include "ks106_rs485.h"
#include "delay.h"
int main(void) {
// Initialize system clock
SystemInit();
// Initialize RS485 communication with KS106
KS106_RS485_Init();
while (1) {
// Read distance from KS106
uint16_t distance = KS106_ReadDistance();
// Display or process the distance value
// For example, print the distance using UART
// printf("Distance: %d mm\r\n", distance);
// Delay before next reading
delay_ms(500);
}
}
```
### 说明
1. **`ks106_rs485.h`**:
- 包含了必要的头文件和宏定义。
- 声明了初始化和读取距离的函数。
2. **`ks106_rs485.c`**:
- 实现了USART1的初始化,用于RS485通信。
- 提供了发送和接收字节的函数。
- 实现了初始化RS485通信和读取距离的函数。
3. **`main.c`**:
- 初始化系统时钟和RS485通信。
- 在主循环中不断读取KS106的距离值,并进行处理(例如打印)。
请确保您的项目中包含了必要的库文件(如`usart.h`和`delay.h`),并且已经正确配置了STM32的硬件资源。希望这些代码对您有所帮助!
阅读全文
相关推荐
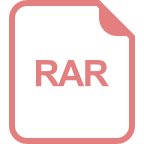
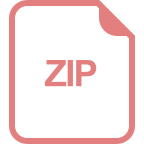
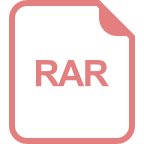















