用c++求一个字符串中大写字母的个数
时间: 2024-12-08 10:24:59 浏览: 18
在C++中,你可以使用标准库函数`std::count_if`配合遍历来计算一个字符串中大写字母的数量。这里是一个简单的示例:
```cpp
#include <iostream>
#include <string>
#include <cctype>
int count_uppercase_letters(const std::string& str) {
int uppercase_count = std::count_if(str.begin(), str.end(), ::isupper);
return uppercase_count;
}
int main() {
std::string input_string = "Hello World!";
int uppercase_letters = count_uppercase_letters(input_string);
std::cout << "The number of uppercase letters is: " << uppercase_letters << '\n';
return 0;
}
```
这个`count_uppercase_letters`函数接受一个字符串作为输入,它通过`isupper`函数检查每个字符是否为大写字母,并使用`std::count_if`统计满足条件的字符数量。
相关问题
c++题目描述 输入一个字符串(不含空白字符),请分别输出该字符串中大写字母、小写字母和数字的个数。 输入描述 输入1行,一个不含空白字符的字符串(字符串长度不超过20)。 输出描述 输出3行,第1行为该字符串中大写字母的个数,第2行为该字符串中小写字母的个数,第3行位该字符串中数字的个数。
好的,你可以使用以下的 C++ 代码来实现这个问题:
```cpp
#include <iostream>
using namespace std;
int main() {
string s;
cin >> s;
int upper = 0, lower = 0, digit = 0;
for (int i = 0; i < s.size(); i++) {
if (s[i] >= 'A' && s[i] <= 'Z') {
upper++;
} else if (s[i] >= 'a' && s[i] <= 'z') {
lower++;
} else if (s[i] >= '0' && s[i] <= '9') {
digit++;
}
}
cout << upper << endl;
cout << lower << endl;
cout << digit << endl;
return 0;
}
```
这个程序首先会读入一个不含空白字符的字符串 `s`,然后我们使用一个循环,依次遍历字符串中的每个字符,如果该字符是大写字母,则将 `upper` 的值加 1;如果该字符是小写字母,则将 `lower` 的值加 1;如果该字符是数字,则将 `digit` 的值加 1。最后,我们分别输出 `upper`、`lower` 和 `digit` 的值,即为字符串中大写字母、小写字母和数字的个数。
希望这个程序可以帮到你!
用c++写一个求出字符串中大写英文字母的个数
好的,下面是 C++ 代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string s;
cout << "请输入字符串:";
getline(cin, s);
int count = 0;
for (char c : s) {
if (isupper(c)) {
count++;
}
}
cout << "大写英文字母的个数为:" << count << endl;
return 0;
}
```
代码中使用了 `string` 类型来表示输入的字符串,使用 `getline` 函数来获取用户输入。然后使用 `isupper` 函数判断每个字符是否为大写英文字母,最后输出大写英文字母的个数。
阅读全文
相关推荐
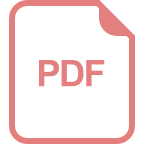
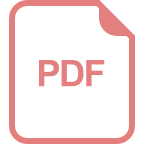
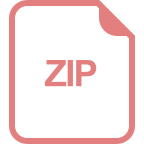













