将这个文档中的1511544070类型的数据使用java的MapReduce转化为2017-12-03这种类型的数据
时间: 2024-09-18 07:17:53 浏览: 68
您提到的“1511544070”这类数据看起来是时间戳(以秒为单位)。要将其转换为日期格式“2017-12-03”,首先需要解析出时间戳,并使用Java中的日期处理类进行格式化。如果要在Hadoop环境中通过MapReduce实现这一目标,可以按照以下步骤设计一个简单的MapReduce程序:
### Mapper部分
Mapper的主要任务是从输入中提取出时间戳字段,并输出键值对<k, v>,这里k可以是任意不影响后续Reducer合并逻辑的标识符,v即为原始的时间戳字符串。
```java
import java.io.IOException;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Mapper;
public class DateConversionMapper extends Mapper<LongWritable, Text, Text, Text> {
protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
// 假设每行只有一个时间戳
String[] parts = value.toString().split(" ");
for (String part : parts) {
if (part.matches("\\d{10}")) { // 匹配长度为10位数的数字
context.write(new Text(part), new Text());
}
}
}
}
```
### Reducer部分
Reducer接收到Mapper传递的所有<v, timeStr>后,只需要负责把每个timeStr从UNIX时间戳转换成所需的日期格式即可。
```java
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Date;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Reducer;
public class DateConversionReducer extends Reducer<Text, Text, Text, Text> {
private static final SimpleDateFormat outputFormat = new SimpleDateFormat("yyyy-MM-dd");
protected void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
long timestamp = Long.parseLong(key.toString());
String dateStr = outputFormat.format(new Date(timestamp * 1000L)); // 转换时乘以1000是因为SimpleDateFormat期望毫秒为单位
context.write(new Text(dateStr), new Text());
}
}
```
以上代码示例展示了如何在Hadoop MapReduce框架下完成从UNIX时间戳到特定日期格式的转换工作。注意实际应用中可能还需要考虑更多细节,比如输入数据的具体结构和形式等。此外,在部署作业前,请确保所有依赖库都正确配置到了Hadoop集群上。
阅读全文
相关推荐
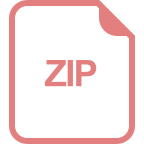
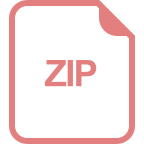
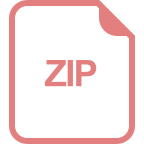
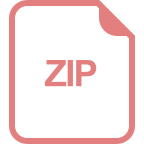
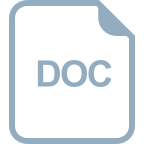
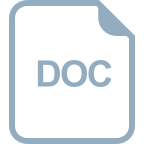
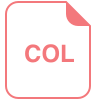
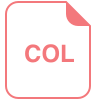
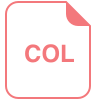
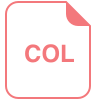
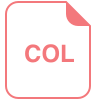
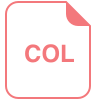
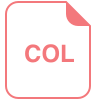
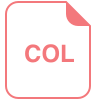
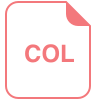
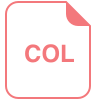
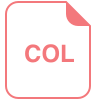


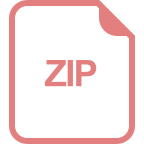