生成一个动态粒子爱心代码
时间: 2024-09-07 09:03:17 浏览: 68
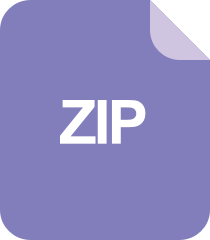
qt/qml粒子系统实现的爱心代码
生成一个动态粒子爱心的代码通常涉及到图形编程,可以选择使用各种图形库,例如在Web前端中常用的HTML5 Canvas结合JavaScript,或者在Python中使用Pygame库等。这里我提供一个简化的示例,使用JavaScript和HTML5的Canvas来绘制一个简单的动态粒子爱心效果。
首先,你需要创建一个HTML文件,并在其中包含一个Canvas元素和相应的JavaScript代码。
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>动态粒子爱心</title>
<style>
body { margin: 0; overflow: hidden; }
canvas { background: #000; display: block; }
</style>
</head>
<body>
<canvas id="heartCanvas"></canvas>
<script>
// 获取Canvas元素并设置宽高
const canvas = document.getElementById('heartCanvas');
const ctx = canvas.getContext('2d');
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
// 粒子数组
const particles = [];
// 粒子类
class Particle {
constructor(x, y) {
this.x = x;
this.y = y;
this.size = Math.random() * 3 + 1; // 粒子大小
this.speed = Math.random() * 1 + 1; // 粒子速度
const angle = Math.random() * Math.PI * 2; // 随机角度
this.vx = Math.cos(angle) * this.speed; // X轴速度分量
this.vy = Math.sin(angle) * this.speed; // Y轴速度分量
}
// 更新粒子位置
update() {
this.x += this.vx;
this.y += this.vy;
}
// 绘制粒子
draw() {
ctx.fillStyle = 'rgba(255, 0, 0, 0.7)'; // 粒子颜色
ctx.beginPath();
ctx.arc(this.x, this.y, this.size, 0, Math.PI * 2);
ctx.fill();
}
}
// 创建粒子
function createHeartShape() {
const x = canvas.width / 2;
const y = canvas.height / 2;
const step = 0.1;
for (let angle = 0; angle < Math.PI * 2; angle += step) {
const r = 16 * Math.pow(Math.sin(angle), 3);
const px = x + r * Math.cos(angle);
const py = y - r * (4 - Math.cos(2 * angle) - Math.cos(4 * angle) - Math.cos(6 * angle) - Math.cos(8 * angle));
particles.push(new Particle(px, py));
}
}
// 动画循环
function animate() {
requestAnimationFrame(animate);
ctx.clearRect(0, 0, canvas.width, canvas.height);
particles.forEach((particle, index) => {
if (particle.x < 0 || particle.x > canvas.width || particle.y < 0 || particle.y > canvas.height) {
particles.splice(index, 1);
} else {
particle.update();
particle.draw();
}
});
}
createHeartShape(); // 初始化粒子形状
animate(); // 开始动画循环
</script>
</body>
</html>
```
这段代码创建了一个全屏的Canvas,并在其中绘制了一个由粒子组成的动态爱心形状。粒子沿着心形轨迹随机生成,并随着时间的推移向上移动,当粒子移动到Canvas边界外时会被移除。
请记得将这段代码保存为HTML文件,并在支持HTML5的浏览器中打开它,以查看效果。
阅读全文
相关推荐
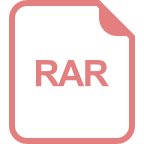
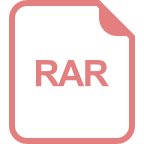















