设顺序表中的数据元素是按值非递减有序排列的,试编写一算法,将x插入到顺序表的适当位置上,以保持顺序表的有序性。
时间: 2024-09-10 09:07:35 浏览: 21
当给定一个已经按照非递减顺序排列的顺序表,并想将新的元素x插入到适当的位,可以采用以下步骤的插入排序算法:
```c
#include <stdio.h>
// 定义顺序表节点结构体
typedef struct {
int data;
struct Node* next;
} Node;
// 用于顺序表的头指针
Node* head = NULL;
// 插入函数
void insertionSortInsert(int x) {
Node* newNode = (Node*)malloc(sizeof(Node)); // 新建节点
newNode->data = x;
newNode->next = NULL;
if (head == NULL) { // 列表为空的情况
head = newNode;
} else {
Node* current = head;
while (current->next != NULL && current->data < x) {
current = current->next;
}
newNode->next = current->next; // 将新节点插入到找到的位置
if (current->next != NULL) {
current->next = newNode;
} else {
current = newNode; // 如果x最大,直接加到列表尾部
}
}
}
int main() {
// 示例插入
insertionSortInsert(5); // 插入5
insertionSortInsert(3); // 插入3
// 打印结果
Node* temp = head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
return 0;
}
相关推荐
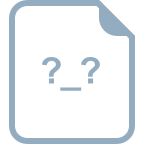
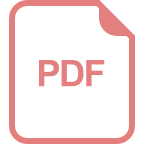















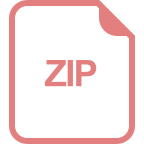