利用搜索算法完成机器人走迷宫c语言
时间: 2023-12-02 10:41:14 浏览: 99
以下是使用C语言实现机器人走迷宫的搜索算法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define MAX_ROW 5
#define MAX_COL 5
struct point {
int row, col;
} queue[512];
int head = 0, tail = 0;
void enqueue(struct point p) {
queue[tail++] = p;
}
struct point dequeue(void) {
return queue[head++];
}
bool is_empty(void) {
return head == tail;
}
bool maze[MAX_ROW][MAX_COL] = {
0, 1, 0, 0, 0,
0, 1, 0, 1, 0,
0, 0, 0, 0, 0,
0, 1, 1, 1, 0,
0, 0, 0, 1, 0,
};
void print_maze(void) {
for (int i = 0; i < MAX_ROW; i++) {
for (int j = 0; j < MAX_COL; j++) {
printf("%d ", maze[i][j]);
}
putchar('\n');
}
printf("*********\n");
}
void visit(int row, int col) {
struct point visit_point = { row, col };
maze[row][col] = 2;
enqueue(visit_point);
}
int main(void) {
struct point p = { 0, 0 };
maze[p.row][p.col] = 2;
enqueue(p);
while (!is_empty()) {
struct point current = dequeue();
if (current.row == MAX_ROW - 1 && current.col == MAX_COL - 1) {
printf("Success!\n");
return 0;
}
if (current.col+1 < MAX_COL && maze[current.row][current.col+1] == 0) {
visit(current.row, current.col+1);
}
if (current.row+1 < MAX_ROW && maze[current.row+1][current.col] == 0) {
visit(current.row+1, current.col);
}
if (current.col-1 >= 0 && maze[current.row][current.col-1] == 0) {
visit(current.row, current.col-1);
}
if (current.row-1 >= 0 && maze[current.row-1][current.col] == 0) {
visit(current.row-1, current.col);
}
print_maze();
}
printf("Failed!\n");
return EXIT_FAILURE;
}
```
阅读全文
相关推荐
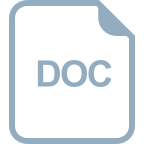
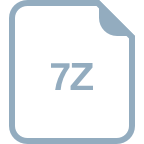
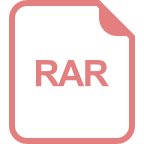
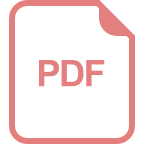
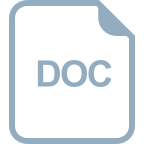
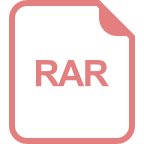
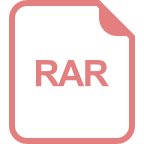
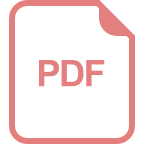
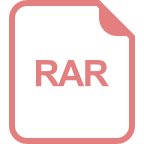
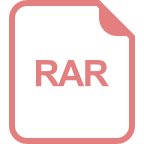
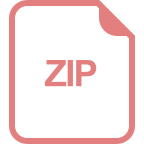
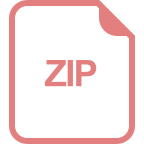
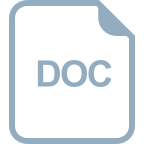
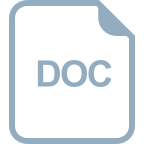
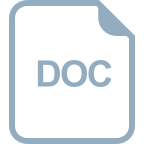