用C加加编写一个程序,单片机发送数据给上位机,同时上位机收到数据后再发送数据给单片机
时间: 2024-12-02 19:19:35 浏览: 0
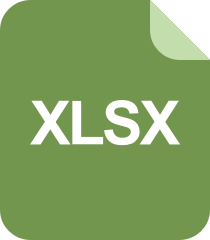
IT老卢-ST加加股票数据-2020年全年历史数据.xlsx
在C语言中编写一个简单的串口通信程序,用于单片机(如Arduino Uno)与上位机(例如PC端的计算机)之间的双向数据交换,通常会涉及到硬件串口配置和软件库的使用,这里我会给出一个基本的伪代码示例。假设我们使用的是UART(通用异步收发传输)。
```c
#include <avr/io.h> // For Arduino-specific UART functions
#include <util/delay.h> // For delay function
// 单片机发送函数
void send_data(int data) {
DDRB |= (1 << PB0); // Set PB0 as output
while (!(UBRR0L == 0)) {}; // Wait for buffer empty
UDR0 = data; // Send data through UART
_delay_ms(1); // Add a short delay to avoid race condition
}
// 上位机接收并回传数据函数
void receive_and_reply(int *received_data) {
char incoming_data;
while (UCSR0A & (1 << RXC0)) { // Wait for new data received
incoming_data = UDR0; // Read received data
*received_data = incoming_data; // Store it in pointer variable
send_data(incoming_data); // Send back the received data
_delay_ms(1); // Short delay before next read
}
}
int main() {
int transmit_data = 42; // Example data to send
// Initialize UART with appropriate baud rate and other settings
setup_UART();
// Start the data exchange loop
while (true) {
send_data(transmit_data);
receive_and_reply(&transmit_data);
}
return 0;
}
```
注意:这个示例是简化版的,并未包含所有错误处理和实际硬件连接细节。在实际应用中,你需要根据具体的硬件平台和串口配置来调整代码。此外,`setup_UART()`、`_delay_ms()`等函数需要你自己实现,通常会涉及到初始化波特率、数据位数、停止位和校验等串口参数。
阅读全文
相关推荐
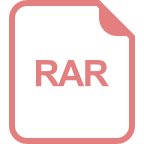















