java 根据文件InputStream下载文件
时间: 2024-09-18 14:08:06 浏览: 42
在Java中,如果你有一个文件的InputStream,可以直接使用FileOutputStream将其复制到本地文件系统,实现下载功能。下面是一个简单的示例:
```java
import java.io.*;
public class FileDownload {
public static void downloadFileByInputStream(InputStream is, String fileName) throws IOException {
FileOutputStream fos = null;
try {
// 创建目标文件
File outputFile = new File(fileName);
// 检查文件是否存在,如果存在则先删除
if (outputFile.exists()) {
outputFile.delete();
}
// 打开目标文件的输出流
fos = new FileOutputStream(outputFile);
// 将InputStream的数据写入到文件中
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = is.read(buffer)) != -1) {
fos.write(buffer, 0, bytesRead);
}
System.out.println("File downloaded successfully!");
} finally {
// 确保关闭资源
if (fos != null) {
fos.close();
is.close();
}
}
}
public static void main(String[] args) {
try {
// 假设你有一个名为inputStream的InputStream
// 实际上,这应该是网络请求或者其他数据来源得到的流
InputStream inputStream = ...;
String destinationFilePath = "path/to/downloaded/file.txt"; // 目标文件路径
downloadFileByInputStream(inputStream, destinationFilePath);
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在这个例子中,`downloadFileByInputStream`函数接收一个InputStream和一个文件名,将InputStream中的数据连续写入到一个新的文件中。确保在操作完成后关闭流以释放资源。
阅读全文
相关推荐






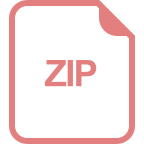











