用C++语言做一个图书管理系统的代码,包括图书信息,查找图书,修改图书信息,插入图书,输出图书,续借图书,删除图书
时间: 2024-12-28 20:34:57 浏览: 0
在C++中设计一个简单的图书管理系统涉及到数据结构、类和文件操作。以下是一个简化的例子,展示了如何创建一个Book类,并提供基本的功能如添加、查询、更新、删除图书以及借阅功能。这里假设我们有一个简单的图书ID,书名和是否可以借阅的信息。
```cpp
#include <iostream>
#include <map>
#include <fstream>
class Book {
public:
int id;
std::string title;
bool isAvailable;
// 构造函数
Book(int id, const std::string& title) : id(id), title(title), isAvailable(true) {}
// 查找图书
static Book* findBook(int id) {
std::ifstream file("library.txt");
if (file.is_open()) {
for (std::string line; std::getline(file, line);) {
auto data = parseLine(line);
if (data.first == id)
return new Book(data.first, data.second);
}
file.close();
}
return nullptr;
}
// 插入图书
void insert() {
std::ofstream file("library.txt", std::ios_base::app);
if (file.is_open())
file << id << "," << title << "," << (isAvailable ? "1" : "0") << "\n";
file.close();
}
// 修改图书信息
void update(const std::string& newTitle, bool newAvailability) {
isAvailable = newAvailability;
title = newTitle;
// 更新文件中的信息,这里简化处理,实际应用需要考虑并发控制
std::ofstream(file("library.txt"), std::ios_base::in | std::ios_base::out);
file.seekg(0, std::ios::beg);
while (!file.eof()) {
std::string line;
getline(file, line);
if (line.find(std::to_string(id)) != std::string::npos) {
file << id << "," << newTitle << "," << (newAvailability ? "1" : "0") << "\n";
break;
}
}
file.close();
}
// 删除图书
void deleteBook() {
// 删除文件中对应的行,同样简化处理,实际应用需要更复杂的逻辑
std::ofstream file("library.txt", std::ios_base::in | std::ios_base::out);
file.seekg(0, std::ios::beg);
std::string line;
while (!file.eof()) {
std::getline(file, line);
if (line.find(std::to_string(id)) != std::string::npos) {
file.seekp(file.tellg() - line.length(), std::ios_base::cur);
file.ignore(numeric_limits<std::streamsize>::max(), '\n');
file.put('\n');
break;
}
}
file.close();
}
private:
static std::pair<int, std::string> parseLine(const std::string& line) {
std::istringstream iss(line);
int id, available;
std::string title;
iss >> id >> title >> available;
return {id, title};
}
};
// 主函数示例,演示如何使用
int main() {
Book book(1, "《计算机程序的构造和解释》");
book.insert();
Book* foundBook = Book::findBook(1);
if (foundBook)
foundBook->update("新书名", false);
book.deleteBook();
return 0;
}
```
请注意,这个例子仅用于教学目的,实际应用中的图书管理系统会更复杂,可能需要数据库支持,错误处理,线程安全等特性。
阅读全文
相关推荐
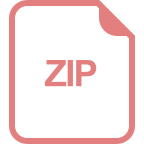
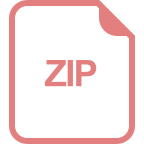
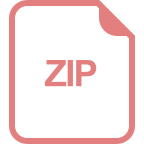
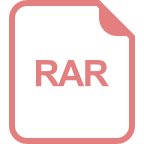
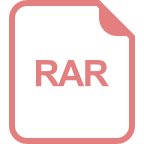
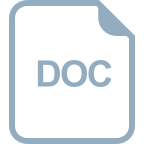
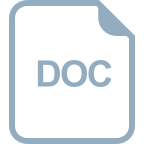
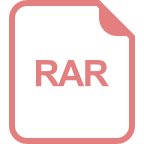
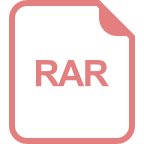
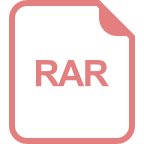
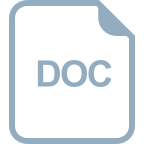
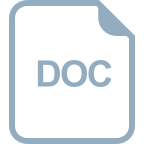
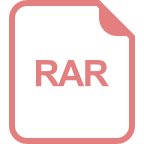
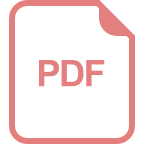
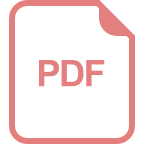
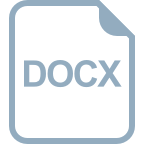
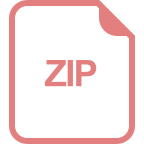
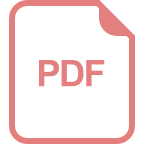