spring boot支持多种图片格式要求:修改代码,使文件上传功能能够支持多种图片格式,包括 JPG、PNG、GIF ,并对不支持的格式返回相应的错误信息。 使用集合保存支持的格式列表,在上传时进行校验。
时间: 2024-11-30 22:29:14 浏览: 2
Spring Boot本身并没有内置针对特定图片格式的检查功能,但是你可以通过一些第三方库如Apache Commons IO或者MultipartFile的mime-type属性来实现这个需求。以下是一个基本的步骤:
1. 首先,你需要在你的控制器或服务类中处理文件上传。可以使用`@RequestParam`注解接收上传的文件,并将其存储到`MultipartFile`对象中。
```java
@PostMapping("/upload")
public ResponseEntity<?> uploadFile(@RequestParam("file") MultipartFile file) {
if (file.isEmpty()) {
return ResponseEntity.badRequest().body("文件不能为空");
}
String allowedTypes = "image/jpeg, image/png, image/gif";
String contentType = file.getContentType();
if (!allowedTypes.contains(contentType)) {
return ResponseEntity.status(HttpStatus.BAD_REQUEST).body("只接受JPG、PNG、GIF格式的图片");
}
// 保存文件的代码...
}
```
2. 在这里,我们检查了`contentType`是否在允许的类型列表中。如果不在,就返回一个错误响应。
3. 如果需要,你可以在上传前验证文件扩展名,因为有时候文件名可能会被篡改,而`contentType`更可靠。这通常涉及到解析文件名并提取扩展名。
4. 为了方便管理,你也可以创建一个枚举或集合来存储所有的允许格式,而不是每次都写字符串:
```java
public enum SupportedImageFormats {
JPG("image/jpeg"),
PNG("image/png"),
GIF("image/gif");
private final String mimeType;
SupportedImageFormats(String mimeType) {
this.mimeType = mimeType;
}
public String getMimeType() {
return mimeType;
}
}
List<SupportedImageFormats> allowedFormats = Arrays.asList(SupportedImageFormats.values());
```
然后在上传方法中替换为:
```java
if (!allowedFormats.stream()
.map(SupportedImageFormats::getMimeType)
.anyMatch(file.getContentType()::equals)) {
//...
}
```
阅读全文
相关推荐
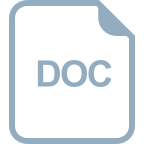
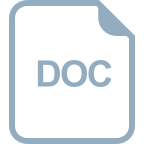
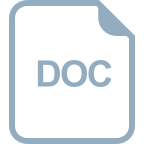

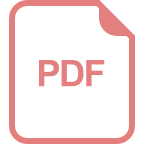
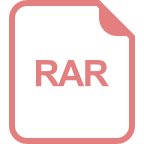
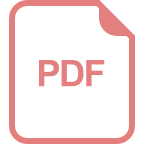
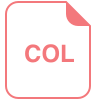
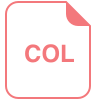


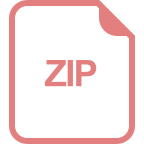
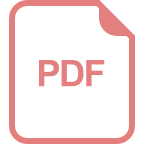
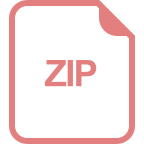
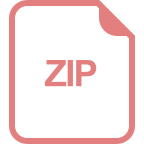
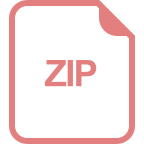
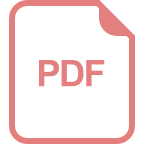
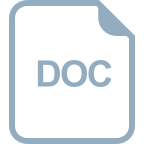
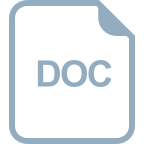