spring boot上传文件只允许图片代码
时间: 2023-06-04 12:03:05 浏览: 147
可以使用以下代码实现只允许上传图片文件:
```
// 设置允许上传的文件类型
List<String> allowedTypes = Arrays.asList("image/jpeg", "image/png", "image/gif");
// 处理文件上传请求
@PostMapping("/upload")
public String handleFileUpload(@RequestParam("file") MultipartFile file) {
if (!allowedTypes.contains(file.getContentType())) {
return "上传文件必须是图片文件";
}
// 文件上传逻辑
...
}
```
以上代码会判断上传的文件类型是否在允许上传的类型列表中,如果不是则返回错误信息。
相关问题
用Spring Boot文件上传写一个实现只能上传图片代码
好的,以下是用 Spring Boot 实现只能上传图片的代码示例:
首先需要添加依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.4</version>
</dependency>
```
然后在控制器中定义上传图片的接口,只允许上传图片类型的文件:
```
import org.apache.commons.io.FilenameUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.util.ResourceUtils;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.util.UUID;
@RestController
public class FileController {
@PostMapping("/uploadImage")
public String handleFileUpload(@RequestParam("file") MultipartFile file) {
String fileName = file.getOriginalFilename();
// 验证是否为图片类型
String extension = FilenameUtils.getExtension(fileName);
if (StringUtils.isBlank(extension) || !extension.matches("^(jpg|jpeg|png|gif)$")) {
return "上传失败,请上传图片文件!";
}
// 生成新的文件名
String newFileName = UUID.randomUUID().toString() + "." + extension;
// 拼接文件路径
File uploadPath;
try {
uploadPath = new File(ResourceUtils.getURL("classpath:").getPath());
if (!uploadPath.exists()) {
uploadPath = new File("");
}
File imgPath = new File(uploadPath.getAbsolutePath(), "/static/upload/img/");
if (!imgPath.exists()) {
imgPath.mkdirs();
}
File dest = new File(imgPath, newFileName);
file.transferTo(dest);
return "/upload/img/" + newFileName;
} catch (FileNotFoundException e) {
e.printStackTrace();
return "上传失败," + e.getMessage();
} catch (IOException e) {
e.printStackTrace();
return "上传失败," + e.getMessage();
}
}
}
```
上面的代码中,首先根据上传的文件名判断是否为图片类型,然后生成新的文件名,将文件保存到指定的路径中,最后返回保存后的文件路径。
请注意,在使用这段代码时,需要在 Spring Boot 项目的 resources 目录下创建 static/upload/img 目录,用于存储上传的图片文件。
文件上传spring boot
在Spring Boot中进行文件上传非常简单。首先,在Spring Boot的配置文件(通常是application.yml或application.properties)中添加以下配置项:
spring.servlet.multipart.enabled=true
spring.servlet.multipart.file-size-threshold=2MB
spring.servlet.multipart.max-file-size=10MB
spring.servlet.multipart.max-request-size=100MB
spring.servlet.multipart.location=your/upload/folder/path
这些配置项允许文件上传并设置了文件大小的阈值和限制。其中,enabled为true表示启用文件上传功能,file-size-threshold表示文件大小的阈值,如果上传的文件大小超过这个阈值,则会被写入磁盘,否则会保存在内存中。max-file-size和max-request-size分别表示单个文件和整个请求的最大文件大小限制。
在具体的代码实现上,你只需要在Controller中添加一个处理文件上传的方法,并使用@RequestParam注解来接收上传的文件。例如:
@PostMapping("/upload")
public String handleFileUpload(@RequestParam("file") MultipartFile file) {
// 处理上传的文件逻辑
// ...
return "success";
}
这个方法会接收名为file的文件参数,并将其保存到指定的位置。你可以在方法体内编写逻辑来处理上传的文件,例如保存到数据库或文件系统中。
需要注意的是,为了使文件上传更加方便,Spring Boot还提供了一些快捷的工具类和注解,例如@RequestPart和@RequestBody等。你可以根据具体的需求选择合适的方式来处理文件上传操作。
总结起来,使用Spring Boot进行文件上传非常简单。你只需要在配置文件中添加相应的配置项,然后在Controller中编写文件上传的处理方法即可。同时,Spring Boot还提供了一些方便的工具类和注解来简化文件上传的操作。
相关推荐
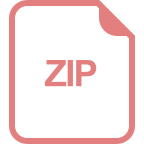
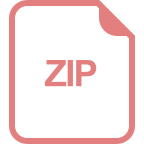
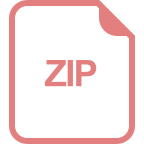












