qt串口通信利用Qmap函数转码控制单片机代码示例 带.h函数及.h函数
时间: 2024-09-14 09:07:36 浏览: 43
Qt中的串口通信通常是通过QSerialPort类来实现的,而不是直接使用QMap函数来处理转码。QMap是Qt提供的一个容器类,用于存储键值对,并不直接用于串口通信。在串口通信中,你可能需要使用QMap来存储一些编码表或映射表,用于转码操作。
下面是一个简单的例子,展示了如何使用Qt进行串口通信,并使用QMap来进行简单的编码转换。
首先是头文件`serial_communication.h`:
```cpp
#ifndef SERIAL_COMMUNICATION_H
#define SERIAL_COMMUNICATION_H
#include <QObject>
#include <QSerialPort>
#include <QMap>
#include <QByteArray>
class SerialCommunication : public QObject
{
Q_OBJECT
public:
explicit SerialCommunication(QObject *parent = nullptr);
bool openPort(const QString &portName, int baudRate);
void closePort();
void sendCommand(const QByteArray &command);
void setEncodingMap(const QMap<QString, QByteArray> &encodingMap);
signals:
void readyRead();
private slots:
void readData();
private:
QSerialPort *serial;
QMap<QString, QByteArray> encodingMap;
};
#endif // SERIAL_COMMUNICATION_H
```
然后是实现文件`serial_communication.cpp`:
```cpp
#include "serial_communication.h"
SerialCommunication::SerialCommunication(QObject *parent) : QObject(parent), serial(new QSerialPort(this))
{
connect(serial, &QSerialPort::readyRead, this, &SerialCommunication::readData);
}
bool SerialCommunication::openPort(const QString &portName, int baudRate)
{
serial->setPortName(portName);
serial->setBaudRate(baudRate);
if (serial->open(QIODevice::ReadWrite)) {
// Here you can set additional serial port configurations if necessary.
return true;
}
return false;
}
void SerialCommunication::closePort()
{
if (serial->isOpen()) {
serial->close();
}
}
void SerialCommunication::sendCommand(const QByteArray &command)
{
serial->write(command);
}
void SerialCommunication::setEncodingMap(const QMap<QString, QByteArray> &encodingMap)
{
this->encodingMap = encodingMap;
}
void SerialCommunication::readData()
{
const QByteArray data = serial->readAll();
// Convert received data using encodingMap
// For example, if you have a map with encodings from strings to byte arrays
QMapIterator<QString, QByteArray> i(encodingMap);
while (i.hasNext()) {
i.next();
if (data.startsWith(i.value())) {
// Handle the received command
break;
}
}
}
```
在使用上述类之前,你需要创建一个`QMap`对象来存储你的转码规则,例如:
```cpp
QMap<QString, QByteArray> encodingMap;
encodingMap.insert("ON", QByteArray::fromHex("4F4E")); // "ON" 对应十六进制 "4F4E"
encodingMap.insert("OFF", QByteArray::fromHex("4F46"));
// ... 添加其他编码规则
SerialCommunication serialComm;
serialComm.setEncodingMap(encodingMap);
```
然后你可以调用`openPort`来打开串口,并使用`sendCommand`发送命令。
阅读全文
相关推荐
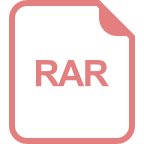
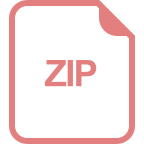
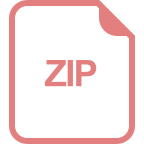














