利用vscode编写python程序,给图片2.png采用中值滤波的方法进行图片去噪
时间: 2024-09-23 11:07:54 浏览: 21
在VSCode中编写Python程序来对图片“2.png”进行中值滤波去噪,你可以使用PIL(Python Imaging Library)库或更现代的OpenCV库。这里是一个简单步骤的概述:
首先,确保已安装所需库:
```bash
pip install pillow opencv-python
```
然后,在VSCode中创建一个新的Python文件(假设名为`image_filter.py`),并导入所需的库:
```python
from PIL import Image
import cv2
```
对于PIL(针对灰度图):
```python
def median_filter_pil(image_path):
image = Image.open(image_path)
width, height = image.size
pixels = list(image.getdata())
filtered_pixels = [pixels[i] for i in range(0, len(pixels), width * height)]
# 使用中值滤波替换像素值
new_filtered_pixels = [median(filter(filtered_pixels[j:j + width])) for j in range(0, len(filtered_pixels), width)]
new_image = Image.new("L", (width, height))
new_image.putdata(new_filtered_pixels)
return new_image
# 中值函数
def median(lst):
lst.sort()
length = len(lst)
if length % 2 == 0:
return (lst[length // 2 - 1] + lst[length // 2]) / 2
else:
return lst[length // 2]
# 图片去噪操作
noisy_image = median_filter_pil('2.png')
noisy_image.save('filtered_2.png')
```
对于OpenCV(处理彩色图像):
```python
import cv2
import numpy as np
def median_filter_cv2(image_path):
img = cv2.imread(image_path, 0) # 读取灰度图像
blurred = cv2.medianBlur(img, 5) # 应用5x5中值滤波器
cv2.imwrite('filtered_2.png', blurred)
median_filter_cv2('2.png')
```
运行这个脚本,它会生成处理后的图片`filtered_2.png`。
相关推荐
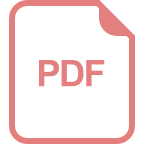
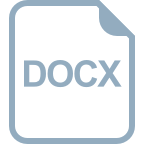
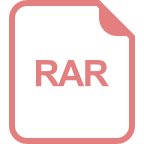














