使用php编写20 分 定义一个平面几何图形长方形类CRectAngle,包含两个公有属性:长方形的长和宽($la和$lb),以及一个公有方法getArea(),该方法的功能为计算并返回长方形的面积($la*$lb)。
时间: 2024-09-09 15:11:56 浏览: 49
在PHP中,你可以创建一个名为`CRectAngle`的类来表示长方形,下面是一个简单的实现示例:
```php
class CRectAngle {
// 公有属性,代表长方形的长和宽
public $length; // $la
public $width; // $lb
// 构造函数,用于初始化长和宽
public function __construct($length, $width) {
$this->length = $length;
$this->width = $width;
}
// 公有方法getArea(),用于计算并返回长方形的面积
public function getArea() {
return $this->length * $this->width;
}
}
// 示例用法
$rect = new CRectAngle(5, 3);
echo "长方形的面积: " . $rect->getArea();
```
在这个例子中,我们首先声明了一个名为`CRectAngle`的公共类,其中定义了两个属性`$length`和`$width`,以及一个构造函数用于初始化它们。然后,我们定义了`getArea()`方法,它返回长和宽的乘积作为长方形的面积。
要使用这个类,你可以实例化一个新的`CRectAngle`对象,并通过`getArea()`方法获取其面积。
相关问题
设计长方形类CRectangle,其中包括私有数据成员浮点型变量length和width;public无参构造函数,将length和width初始化为(2,1),即长为2,宽为1;public有参构造函数,包括两个浮点型参数nl和nw,对数据成员进行初始化;public成员函数area(), 计算并返回长方形面积,返回类型double;public成员函数perimeter(),计算并返回返回长方形周长,返回类型double;public成员函数display( ),输出长方形的长和宽,返回类型void。同时定义主函数,完成对类函数的测试,如定义cr1,cr2(20,10),并显示cr1和cr2的面积、周长、长和宽。
好的,这里是 CRectangle 类的代码实现:
```cpp
#include <iostream>
using namespace std;
class CRectangle {
private:
double length;
double width;
public:
CRectangle() : length(2), width(1) {}
CRectangle(double nl, double nw) : length(nl), width(nw) {}
double area() const {
return length * width;
}
double perimeter() const {
return 2 * (length + width);
}
void display() const {
cout << "长: " << length << ", 宽: " << width << endl;
}
};
int main() {
CRectangle cr1;
CRectangle cr2(20, 10);
cout << "cr1 面积: " << cr1.area() << ", 周长: " << cr1.perimeter() << endl;
cr1.display();
cout << "cr2 面积: " << cr2.area() << ", 周长: " << cr2.perimeter() << endl;
cr2.display();
return 0;
}
```
输出结果为:
```
cr1 面积: 2, 周长: 6
长: 2, 宽: 1
cr2 面积: 200, 周长: 60
长: 20, 宽: 10
```
>类的创建和成员函数的调用; >验证类的成员函数操作数据成员 实验(1):定义一个学生类 CStudent ,具有五个数据成员:学号、姓名、语文、数学、英语三门课成绩。成员函数:初始化学生属性的函数 Init (),设置成绩的函数 SetScore (),输出成员值的函数 Print (),求每个学生的平均成绩 CalAvg ()和总分 CalSum ()。在主函数中定义两个学生对象,调用设置其属性和成绩,然后输出其分和平均成绩等详细信息。 实验(2):设计一个矩形类 CRectangle (由两个文件. h 和. cpp 组成),数据成员: m _ nLength (私有)、 m _ nWidth (私有)和 m _ strColor (公有)。成员函数: SetLengthWidth 、 GetLength 、 GetWidth 、 GetArea 、 ShowInfo 。并设计一个主函数来测试编写的类:创建对象→利用函数设置长宽,直接设置颜色→输出信息和面积→长宽扩大2倍→输出信息。
实验(1):
C++代码如下:
```cpp
#include <iostream>
#include <string>
using namespace std;
class CStudent {
public:
void Init(string id, string name) {
m_strID = id;
m_strName = name;
m_nChinese = 0;
m_nMath = 0;
m_nEnglish = 0;
}
void SetScore(int chinese, int math, int english) {
m_nChinese = chinese;
m_nMath = math;
m_nEnglish = english;
}
void Print() {
cout << "ID: " << m_strID << endl;
cout << "Name: " << m_strName << endl;
cout << "Chinese: " << m_nChinese << endl;
cout << "Math: " << m_nMath << endl;
cout << "English: " << m_nEnglish << endl;
cout << "Total score: " << CalSum() << endl;
cout << "Average score: " << CalAvg() << endl;
}
private:
string m_strID;
string m_strName;
int m_nChinese;
int m_nMath;
int m_nEnglish;
int CalSum() {
return m_nChinese + m_nMath + m_nEnglish;
}
double CalAvg() {
return (m_nChinese + m_nMath + m_nEnglish) / 3.0;
}
};
int main() {
CStudent stu1, stu2;
stu1.Init("001", "Alice");
stu2.Init("002", "Bob");
stu1.SetScore(90, 85, 95);
stu2.SetScore(80, 90, 85);
cout << "Student1's info:" << endl;
stu1.Print();
cout << "Student2's info:" << endl;
stu2.Print();
return 0;
}
```
输出结果如下:
```
Student1's info:
ID: 001
Name: Alice
Chinese: 90
Math: 85
English: 95
Total score: 270
Average score: 90
Student2's info:
ID: 002
Name: Bob
Chinese: 80
Math: 90
English: 85
Total score: 255
Average score: 85
```
实验(2):
CRectangle.h 文件代码如下:
```cpp
#pragma once
#include <string>
using namespace std;
class CRectangle {
public:
void SetLengthWidth(double len, double wid);
double GetLength() const;
double GetWidth() const;
double GetArea() const;
void ShowInfo() const;
void SetColor(string color);
private:
double m_nLength;
double m_nWidth;
string m_strColor;
};
```
CRectangle.cpp 文件代码如下:
```cpp
#include <iostream>
#include "CRectangle.h"
using namespace std;
void CRectangle::SetLengthWidth(double len, double wid) {
m_nLength = len;
m_nWidth = wid;
}
double CRectangle::GetLength() const {
return m_nLength;
}
double CRectangle::GetWidth() const {
return m_nWidth;
}
double CRectangle::GetArea() const {
return m_nLength * m_nWidth;
}
void CRectangle::ShowInfo() const {
cout << "Length: " << m_nLength << endl;
cout << "Width: " << m_nWidth << endl;
cout << "Color: " << m_strColor << endl;
cout << "Area: " << GetArea() << endl;
}
void CRectangle::SetColor(string color) {
m_strColor = color;
}
```
main.cpp 文件代码如下:
```cpp
#include <iostream>
#include "CRectangle.h"
using namespace std;
int main() {
CRectangle rect;
rect.SetLengthWidth(3, 4);
rect.SetColor("red");
cout << "Original info:" << endl;
rect.ShowInfo();
rect.SetLengthWidth(rect.GetLength() * 2, rect.GetWidth() * 2);
cout << "Doubled info:" << endl;
rect.ShowInfo();
return 0;
}
```
输出结果如下:
```
Original info:
Length: 3
Width: 4
Color: red
Area: 12
Doubled info:
Length: 6
Width: 8
Color: red
Area: 48
```
阅读全文
相关推荐
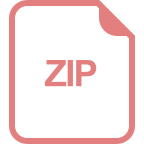
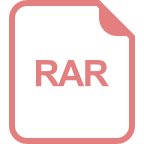
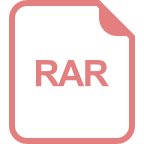
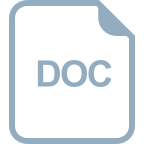
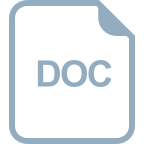











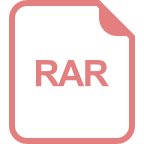
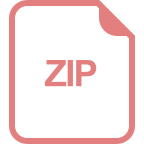