linux下删除某个文件的c代码
时间: 2024-10-01 14:02:00 浏览: 35
在Linux下,如果你想通过C语言编写代码来删除某个文件,你可以使用POSIX标准提供的` unlink()` 函数。这个函数用于删除指定路径下的文件。以下是简单的示例:
```c
#include <stdio.h>
#include <unistd.h>
#include <sys/stat.h>
#include <errno.h>
int main(int argc, char *argv[]) {
if (argc != 2) {
fprintf(stderr, "Usage: %s <file_path>\n", argv[0]);
return 1;
}
char *filePath = argv[1];
struct stat fileStat;
if (stat(filePath, &fileStat) == -1) { // 检查文件是否存在
perror("Error checking file existence");
return 1;
} else if (!S_ISREG(fileStat.st_mode)) { // 判断是否普通文件
perror("Error: Not a regular file");
return 1;
}
if (unlink(filePath) == 0) { // 删除文件
printf("File '%s' deleted successfully.\n", filePath);
} else {
perror("Error deleting file");
return 1;
}
return 0;
}
```
在这个例子中,程序接受一个命令行参数作为文件路径,首先检查文件是否存在且是否是普通文件,然后尝试删除它。如果删除失败,会打印错误信息。
阅读全文
相关推荐
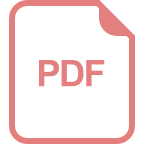
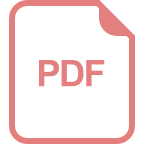
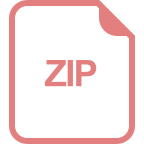















