成功示例:{"code": 200, "message": "Sucess" } 失败示例: { "data": null, "code": 500, "message": "system error"} 怎么在输出的时候封装好code和message
时间: 2024-01-23 11:01:51 浏览: 135
你可以使用字典来封装code和message,然后将其转换为JSON格式输出。以下是一个示例代码:
```python
success_response = {"code": 200, "message": "Sucess"}
failure_response = {"code": 500, "message": "system error"}
import json
# 输出成功示例
print(json.dumps(success_response)) # 输出:{"code": 200, "message": "Sucess"}
# 输出失败示例
print(json.dumps(failure_response)) # 输出:{"code": 500, "message": "system error"}
```
相关问题
syntax = "proto3"; package rss.perception; // import "image_raw.proto"; // road side peception service service PerceptionService { rpc execute (stream ImageRawData) returns (Response) {}; } // meta info of image message ImageMeta { string request_id=1; uint32 seq=2; uint64 time_stamp=3; string device_ip=4; } // raw image data to submit message ImageRawData { ImageMeta Header=1; bytes data=2; } // result of RPC message Response { string request_id=1; int32 code=2; // 200 sucess }请基于上面的proto文件用C++实现一个gRPC的client和server,并给出测试demo
这里是一个基于上面的proto文件使用C++实现的gRPC的client和server,并附带一个测试demo。
首先,需要安装gRPC和Protocol Buffers库。可以根据自己的操作系统和编译器选择对应的安装方法。
接下来,根据proto文件生成对应的C++代码。可以使用以下命令:
```shell
protoc --grpc_out=. --cpp_out=. --proto_path=. your_proto_file.proto
```
这将在当前目录下生成`your_proto_file.pb.h`和`your_proto_file.pb.cc`两个文件,其中包含了生成的代码。
接下来,我们可以编写一个gRPC的server和client,实现上述的服务:
```cpp
// server.cc
#include <iostream>
#include <memory>
#include <string>
#include <thread>
#include <grpcpp/grpcpp.h>
#include "your_proto_file.pb.h" // 替换成你的proto文件名
using grpc::Server;
using grpc::ServerBuilder;
using grpc::ServerContext;
using grpc::Status;
using rss::perception::ImageMeta;
using rss::perception::ImageRawData;
using rss::perception::PerceptionService;
using rss::perception::Response;
class PerceptionServiceImpl final : public PerceptionService::Service {
public:
Status execute(ServerContext* context, grpc::ServerReader<ImageRawData>* reader, Response* response) override {
ImageRawData image_raw_data;
while (reader->Read(&image_raw_data)) {
// 在这里对图像数据进行处理,并将结果写入response中
}
return Status::OK;
}
};
void RunServer() {
std::string server_address("0.0.0.0:50051");
PerceptionServiceImpl service;
ServerBuilder builder;
builder.AddListeningPort(server_address, grpc::InsecureServerCredentials());
builder.RegisterService(&service);
std::unique_ptr<Server> server(builder.BuildAndStart());
std::cout << "Server listening on " << server_address << std::endl;
server->Wait();
}
int main(int argc, char** argv) {
RunServer();
return 0;
}
```
上面的代码实现了一个名为`PerceptionServiceImpl`的gRPC服务类,其中的`execute`方法接收一个图像数据的流并对其进行处理,并将处理结果写入响应中。`RunServer`函数用于启动服务器。
接下来,我们编写一个gRPC的client,向上述的服务发送请求,并打印出响应:
```cpp
// client.cc
#include <iostream>
#include <memory>
#include <string>
#include <thread>
#include <grpcpp/grpcpp.h>
#include "your_proto_file.pb.h" // 替换成你的proto文件名
using grpc::Channel;
using grpc::ClientContext;
using grpc::ClientReader;
using grpc::Status;
using rss::perception::ImageMeta;
using rss::perception::ImageRawData;
using rss::perception::PerceptionService;
using rss::perception::Response;
class PerceptionServiceClient {
public:
PerceptionServiceClient(std::shared_ptr<Channel> channel)
: stub_(PerceptionService::NewStub(channel)) {}
void execute(const std::string& request_id, const std::string& data) {
Response response;
ImageMeta image_meta;
image_meta.set_request_id(request_id);
image_meta.set_seq(0);
image_meta.set_time_stamp(0);
image_meta.set_device_ip("");
ImageRawData image_raw_data;
image_raw_data.set_allocated_header(&image_meta);
image_raw_data.set_data(data);
ClientContext context;
std::unique_ptr<ClientReader<ImageRawData>> reader(stub_->execute(&context, image_raw_data));
while (reader->Read(&response)) {
// 在这里对响应进行处理
}
Status status = reader->Finish();
if (status.ok()) {
std::cout << "Request successful." << std::endl;
} else {
std::cout << "Request failed with status code: " << status.error_code() << std::endl;
}
}
private:
std::unique_ptr<PerceptionService::Stub> stub_;
};
int main(int argc, char** argv) {
PerceptionServiceClient client(grpc::CreateChannel("localhost:50051", grpc::InsecureChannelCredentials()));
client.execute("request_id_1", "raw_image_data_1");
return 0;
}
```
上面的代码实现了一个名为`PerceptionServiceClient`的gRPC客户端类,其中的`execute`方法用于向服务端发送请求,并接收并处理响应。
最后,我们可以编写一个测试demo,将上述的server和client放在一起运行:
```cpp
// test.cc
#include <thread>
#include "server.cc"
#include "client.cc"
int main(int argc, char** argv) {
std::thread server_thread(RunServer);
std::this_thread::sleep_for(std::chrono::milliseconds(1000)); // 等待服务器启动
PerceptionServiceClient client(grpc::CreateChannel("localhost:50051", grpc::InsecureChannelCredentials()));
client.execute("request_id_1", "raw_image_data_1");
server_thread.join();
return 0;
}
```
上面的代码启动了一个服务器和一个客户端,并向服务器发送了一个请求。可以根据自己的需要修改请求参数和处理逻辑。
我的初始化cornerstonecore.init().then(function(){ // 初始化成功后执行的代码 console.log('init sucess'); }).catch(function(error){ // 初始化失败后执行的代码 console.log('init faild'); });控制台成功输出了init sucess信息,但在浏览器中的consloe还是出现了Uncaught Error: @cornerstonejs/core is not initialized, run init() first at new RenderingEngine (RenderingEngine.js:79:19) at eval (app.js:124:25) at ./src/app.js (app.js:5413:1) at __webpack_require__ (app.js:5738:33) at app.js:6837:37 at app.js:6839:12这是为什么
这个问题可能是因为你在初始化之后立即使用了Cornerstone的某些功能,但是这些功能需要一些时间来加载和准备。你可以使用`cornerstoneWADOImageLoader`来确保所有图像加载器都已准备就绪,然后再使用Cornerstone的渲染引擎。
以下是一个使用`cornerstoneWADOImageLoader`的例子:
```
import * as cornerstone from 'cornerstone-core';
import * as cornerstoneWADOImageLoader from 'cornerstone-wado-image-loader';
// 初始化Cornerstone和图像加载器
cornerstoneWADOImageLoader.external.cornerstone = cornerstone;
cornerstoneWADOImageLoader.webWorkerManager.initialize({
maxWebWorkers: navigator.hardwareConcurrency || 1,
startWebWorkersOnDemand: true,
taskConfiguration: {
decodeTask: {
loadCodecsOnStartup: true,
initializeCodecsOnStartup: false,
codecsPath: '/cornerstone/codecs/'
}
}
});
// 等待图像加载器准备就绪
cornerstoneWADOImageLoader.webWorkerManager.onInitialized(function(){
// 执行渲染操作
cornerstone.renderWebGL(canvas, imageData, cornerstone.getDefaultViewport(image, canvas));
});
```
在这个例子中,我们使用`cornerstoneWADOImageLoader`来初始化Cornerstone和图像加载器,并等待图像加载器准备就绪之后再执行渲染操作。你可以根据你的需求修改这个例子,并确保在使用Cornerstone之前等待所有必要的组件都已准备就绪。
阅读全文
相关推荐
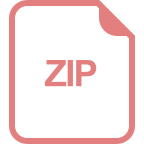
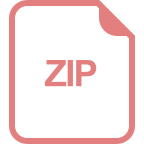
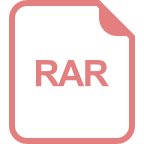
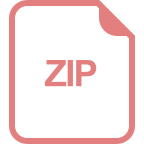
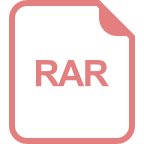
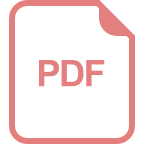
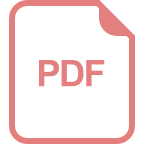






