js获取当前时间的字符串
时间: 2023-11-22 11:47:19 浏览: 132
可以使用如下代码获取当前时间的字符串:
```javascript
function getDate() {
var date = new Date();
var month = date.getMonth() + 1;
var strDate = date.getDate();
if (month >= 1 && month <= 9) {
month = "0" + month;
}
if (strDate >= 0 && strDate <= 9) {
strDate = "0" + strDate;
}
var currentDate = date.getFullYear() + '年' + month + '月' + strDate + '日';
return currentDate;
}
```
调用该函数即可获取当前时间的字符串。
相关问题
js 获取当前日期字符串
要获取当前日期字符串,你可以使用 JavaScript 中的 Date 对象和相应的方法。以下是获取当前日期字符串的一种常见方式:
```javascript
const currentDate = new Date();
const year = currentDate.getFullYear();
const month = String(currentDate.getMonth() + 1).padStart(2, '0');
const day = String(currentDate.getDate()).padStart(2, '0');
const dateString = `${year}-${month}-${day}`;
console.log(dateString);
```
这段代码将获取当前日期,并将其格式化为 'YYYY-MM-DD' 的字符串。例如,如果今天是2022年9月15日,则输出结果为 '2022-09-15'。你可以根据需要自定义日期字符串的格式。
js 获取时间转字符串
在JavaScript中,获取当前时间并将其转换成字符串通常使用`Date`对象及其内置的方法。以下是几种常见的方式:
1. **当前日期和时间:**
```javascript
let now = new Date();
```
2. **格式化为"年月日 时分秒"样式:**
```javascript
let formattedTime = now.toLocaleString('default', {timeZone: 'UTC', year: 'numeric', month: 'long', day: 'numeric', hour: 'numeric', minute: 'numeric', second: 'numeric'});
```
3. **仅日期:**
```javascript
let dateStr = now.getFullYear() + '-' + (now.getMonth()+1) + '-' + now.getDate();
```
4. **仅时间(小时、分钟、秒):**
```javascript
let timeStr = now.getHours() + ':' + now.getMinutes() + ':' + now.getSeconds();
```
如果你想自定义格式,可以使用`Intl.DateTimeFormat`构造函数:
```javascript
let formatter = new Intl.DateTimeFormat('en-US', {
year: 'numeric',
month: 'long',
day: 'numeric',
hour: '2-digit',
minute: '2-digit',
});
let customTimeStr = formatter.format(now);
```
阅读全文
相关推荐



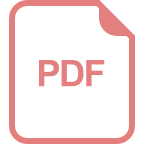
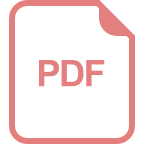







