有5 个学生,每个学生的数据包括学号、姓名、3 门课的成绩。从键盘输入5个学生数据,要求输出每个学生的各项数据及3 门课平均成绩,以及最高平均分的学生各项数据(包括学号、姓名、3 门课的成绩、平均分数) 用 c 语言
时间: 2024-09-26 13:10:23 浏览: 138
在 C 语言中,你可以使用结构体来存储学生的信息,数组来存储所有学生,然后通过循环遍历输入的数据并计算平均成绩。以下是一个简单的示例程序:
```c
#include <stdio.h>
#include <string.h>
// 定义学生结构体
typedef struct {
int student_id;
char name[50];
int grade1, grade2, grade3;
} Student;
int main() {
// 定义最大学生数
const int MAX_STUDENTS = 5;
Student students[MAX_STUDENTS];
for (int i = 0; i < MAX_STUDENTS; i++) {
printf("请输入第 %d 学生的信息 (学号, 姓名, 课程1成绩, 课程2成绩, 课程3成绩): ", i + 1);
scanf("%d%s%d%d%d", &students[i].student_id, students[i].name, &students[i].grade1, &students[i].grade2, &students[i].grade3);
// 计算平均成绩
float average_grade = (float)(students[i].grade1 + students[i].grade2 + students[i].grade3) / 3;
printf("学生 %d - %s 的成绩: 学号:%d, 成绩1:%d, 成绩2:%d, 成绩3:%d, 平均成绩:%f\n",
i + 1, students[i].name, students[i].student_id, students[i].grade1, students[i].grade2, students[i].grade3, average_grade);
}
// 找出最高平均分的学生
float max_average = 0;
int max_student_index = -1;
for (int i = 0; i < MAX_STUDENTS; i++) {
if (average_grade > max_average) {
max_average = average_grade;
max_student_index = i;
}
}
if (max_student_index != -1) {
printf("\n最高平均分的学生信息: 学号:%d, 姓名:%s, 成绩: %d, %d, %d, 平均成绩:%f\n",
students[max_student_index].student_id, students[max_student_index].name,
students[max_student_index].grade1, students[max_student_index].grade2, students[max_student_index].grade3, max_average);
} else {
printf("\n没有找到平均分最高的学生。\n");
}
return 0;
}
```
运行这个程序时,用户会按照提示逐个输入学生的信息,然后程序会计算每个学生的平均成绩,并找出最高平均分的学生。注意,此代码未处理输入验证,实际应用中需要加入错误检查。
阅读全文
相关推荐

















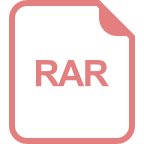