二次线性插值python
时间: 2024-02-15 08:00:47 浏览: 26
二次线性插值是一种插值方法,用于在已知数据点之间估计未知点的值。在Python中,可以使用SciPy库中的interp2d函数来实现二次线性插值。
首先,确保已经安装了SciPy库。可以使用以下命令进行安装:
```
pip install scipy
```
接下来,可以使用以下代码示例来进行二次线性插值:
```python
import numpy as np
from scipy.interpolate import interp2d
# 定义已知数据点的坐标和值
x = np.array([1, 2, 3])
y = np.array([1, 2, 3])
z = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# 创建二次线性插值函数
f = interp2d(x, y, z, kind='quintic')
# 在未知点处进行插值
x_new = 1.5
y_new = 2.5
z_new = f(x_new, y_new)
print(z_new)
```
在上述代码中,首先定义了已知数据点的坐标和值。然后,使用interp2d函数创建了一个二次线性插值函数。最后,通过调用插值函数并传入未知点的坐标,可以得到该点的估计值。
注意,interp2d函数中的kind参数指定了插值方法,这里使用了'quintic'表示二次线性插值。
相关问题
python opencv 二次线性插值
二次线性插值是一种在图像处理中常用的插值方法,可以用于对图像进行缩放、旋转等操作。在 Python 中,可以使用 OpenCV 库来实现二次线性插值。
下面是一个实现二次线性插值的示例代码:
```python
import cv2
def bilinear_interpolation(img, x, y):
# 取整数部分和小数部分
x1, y1 = int(x), int(y)
x2, y2 = x1+1, y1+1
r1 = (x2-x)*(y2-y)
r2 = (x-x1)*(y2-y)
r3 = (x2-x)*(y-y1)
r4 = (x-x1)*(y-y1)
# 根据公式进行插值计算
result = img[y1,x1]*r1 + img[y1,x2]*r2 + img[y2,x1]*r3 + img[y2,x2]*r4
return result
def resize_bilinear(img, dst_size):
height, width = img.shape[:2]
dst_height, dst_width = dst_size
dst_img = np.zeros((dst_height, dst_width, 3), dtype=np.uint8)
scale_x, scale_y = float(width) / dst_width, float(height) / dst_height
for dst_y in range(dst_height):
for dst_x in range(dst_width):
# 计算原图上对应的坐标
src_x = (dst_x + 0.5) * scale_x - 0.5
src_y = (dst_y + 0.5) * scale_y - 0.5
if src_x < 0 or src_x >= width or src_y < 0 or src_y >= height:
continue
# 进行插值计算
dst_img[dst_y, dst_x] = bilinear_interpolation(img, src_x, src_y)
return dst_img
```
其中,`bilinear_interpolation` 函数实现了二次线性插值的算法,`resize_bilinear` 函数则是用于对图像进行缩放的函数。
使用示例:
```python
import cv2
# 读取图像
img = cv2.imread('test.jpg')
# 缩放图像
dst_size = (int(img.shape[1] * 0.5), int(img.shape[0] * 0.5))
dst_img = resize_bilinear(img, dst_size)
# 显示图像
cv2.imshow('Original', img)
cv2.imshow('Resized', dst_img)
cv2.waitKey(0)
```
python生成气象二次线性插值图
生成气象二次线性插值图可以使用Python中的scipy库来实现。以下是一个简单的示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
from scipy.interpolate import griddata
# 构造数据
x = np.random.rand(100) * 10
y = np.random.rand(100) * 10
z = np.sin(x) + np.cos(y)
# 定义网格点
xi = np.linspace(0, 10, 100)
yi = np.linspace(0, 10, 100)
xi, yi = np.meshgrid(xi, yi)
# 进行二次线性插值
zi = griddata((x, y), z, (xi, yi), method='cubic')
# 绘制插值图
plt.contourf(xi, yi, zi, cmap='RdYlBu')
plt.colorbar()
plt.show()
```
这段代码首先构造了一些随机的数据,然后定义了一个网格点,并使用griddata函数进行二次线性插值。最后,使用contourf函数绘制插值图。你可以根据自己的数据进行调整。
相关推荐
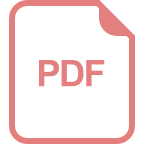
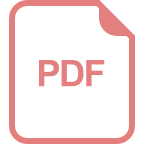
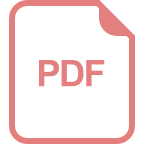












