单向循环链表的基本操作c++代码写出来
时间: 2024-11-03 22:20:42 浏览: 24
单向循环链表是一种特殊的链表结构,它的最后一个节点指向第一个节点,形成一个环。在C++中,我们可以创建一个Node结构体表示节点,并实现一些基本操作,如插入、删除和遍历。以下是简单的实现:
```cpp
#include <iostream>
// 定义链表节点结构
struct Node {
int data;
Node* next; // 指向前一个节点,构成循环
Node(int value) : data(value), next(this) {} // 构造函数设置next指针为自身
};
// 插入节点到链表头部
void insertAtHead(Node*& head, int value) {
Node* newNode = new Node(value);
newNode->next = head;
head = newNode;
}
// 插入节点到链表尾部(这里是循环链表,所以实际上是头部)
void insertAtTail(Node*& head, int value) {
if (!head) {
head = new Node(value);
head->next = head;
} else {
Node* current = head;
while (current->next != head) {
current = current->next;
}
current->next = new Node(value);
current->next->next = head;
}
}
// 删除给定值的节点
void deleteNode(Node*& head, int value) {
if (!head) return;
if (head->data == value) {
Node* temp = head;
head = head->next;
delete temp;
return;
}
Node* current = head;
while (current->next != head && current->data != value) {
current = current->next;
}
if (current->data == value) {
current->next = head;
delete current;
}
}
// 遍历并打印链表
void printList(Node* head) {
if (!head) return;
Node* current = head;
do {
std::cout << current->data << " ";
current = current->next;
} while (current != head);
std::cout << std::endl;
}
int main() {
Node* head = nullptr;
// 创建并初始化循环链表...
insertAtTail(head, 5); // 示例插入节点
printList(head); // 打印链表
deleteNode(head, 5); // 删除节点
printList(head); // 再次打印链表
return 0;
}
```
阅读全文
相关推荐




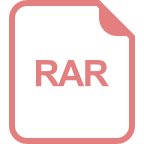











