python如何用socket套接字,来让客户端给服务器端传一个可执行文件,并且能保证服务器端接受完可以直接执行
时间: 2024-09-15 13:12:16 浏览: 41
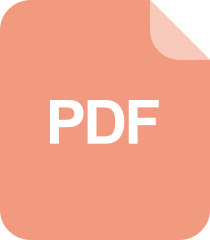
socket网络程序设计实验二,客户端向服务器端发送并接收反馈消息(基于python3.7、PyQt5)
在Python中,你可以使用socket库来创建一个简单的客户端-服务器模型,让客户端发送一个可执行文件到服务器并允许服务器端接收并执行。这里我们将演示一个基础流程:
首先,你需要安装`paramiko`库,它提供了一种安全的方式来传输二进制数据,例如文件,因为Python的内置socket模块默认不支持直接处理二进制文件。
```bash
pip install paramiko
```
**服务器端(server.py):**
```python
import socket
from os import system
def handle_client(client_socket):
# 接收文件大小信息
file_size_str = client_socket.recv(1024).decode()
# 获取整数大小
file_size = int(file_size_str)
# 创建内存缓冲区来接收文件
in_memory_file = bytearray(file_size)
# 逐步接收文件内容
received = 0
while received < file_size:
data = client_socket.recv(min(file_size - received, 1024))
if not data: break
in_memory_file[received:received + len(data)] = data
received += len(data)
# 将接收的字节流保存到临时文件
with open("temp_file", "wb") as temp_file:
temp_file.write(in_memory_file)
# 执行文件
try:
system(f"chmod +x temp_file")
system("./temp_file") # 假设文件名是"temp_file"
except Exception as e:
print(f"Error executing file: {e}")
if __name__ == "__main__":
server_address = ('', 8000) # IP地址和端口号
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind(server_address)
server_socket.listen(1)
print(f"Server started on port {server_address[1]}")
while True:
client_connection, client_address = server_socket.accept()
print(f"Accepted connection from {client_address}")
handle_client(client_connection)
client_connection.close()
```
**客户端(client.py):**
```python
import socket
import paramiko
def send_file_to_server(host, port, file_path):
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
transport = paramiko.Transport((host, port))
transport.connect(username='your_username', password='your_password') # 替换为实际用户名和密码
sftp = paramiko.SFTPClient.from_transport(transport)
sftp.put(file_path, "temp_file") # 发送文件到服务器
sftp.close()
transport.close()
# 使用示例
send_file_to_server('localhost', 8000, 'path/to/your/executable')
```
**注意事项:**
- 这里假设服务器端运行的是Linux环境,因为`chmod`和`./`命令用于修改权限和执行文件。如果服务器不是Linux,需要相应调整执行文件的方式。
- 客户端需要知道服务器的IP地址、端口、用户名和密码才能连接。
- 对于安全性考虑,尽量避免在生产环境中使用明文存储的密码。可以考虑使用SSH密钥对或HTTPS加密通信。
阅读全文
相关推荐
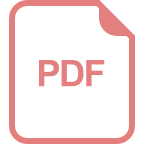
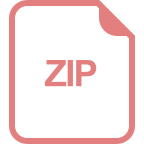
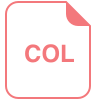
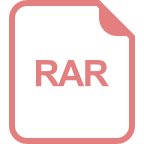
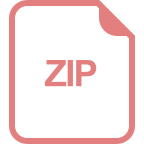
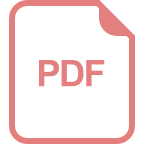
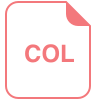
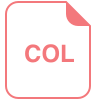
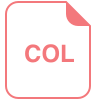
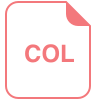
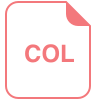
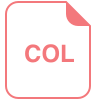
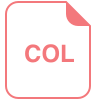
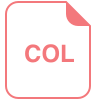
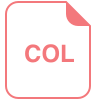
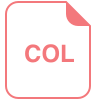
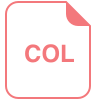