给我一段用dfs或bfs生成最短路径的c++代码
时间: 2024-10-20 09:13:15 浏览: 23
在C++中,我们可以使用深度优先搜索(DFS)或广度优先搜索(BFS)算法来解决图中的最短路径问题。这里我将展示如何使用BFS算法,因为它通常用于求解无权重边的情况下的最短路径。对于有向图,假设我们有一个邻接矩阵表示,我们可以利用`std::queue`来实现BFS。
```cpp
#include <iostream>
#include <vector>
#include <queue>
// 定义图的节点结构
struct Node {
int id;
std::vector<int> neighbors; // 存储邻居节点ID
};
// 使用队列实现BFS
bool bfs(Node graph[], int start, int end) {
std::queue<Node*> queue;
std::vector<bool> visited(graph.length, false); // 标记节点是否已访问
// 将起始点加入队列并标记为已访问
queue.push(&graph[start]);
visited[start] = true;
while (!queue.empty()) {
Node* current = queue.front();
queue.pop();
if (current->id == end) {
return true; // 找到目标节点,返回true
}
for (int neighbor : current->neighbors) {
if (!visited[neighbor]) {
queue.push(&graph[neighbor]);
visited[neighbor] = true;
}
}
}
return false; // 没有找到最短路径,返回false
}
int main() {
// 初始化一个示例图
Node graph[] = {{0, 1}, {0, 2}, {1, 3}, {2, 3}};
int num_nodes = sizeof(graph) / sizeof(graph[0]);
// 调用函数并检查从0到3是否有最短路径
if (bfs(graph, 0, 3)) {
std::cout << "Shortest path exists from 0 to 3.\n";
} else {
std::cout << "No shortest path from 0 to 3.\n";
}
return 0;
}
```
阅读全文
相关推荐
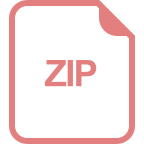
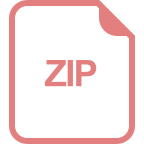
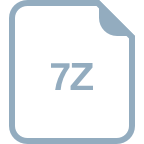

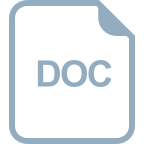
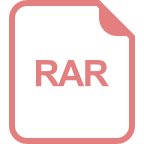
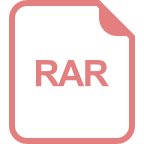
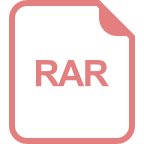
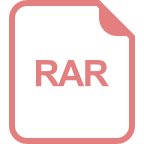
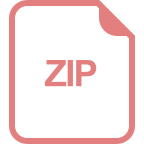
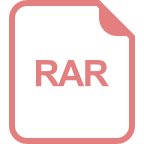
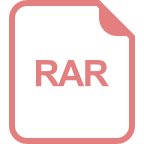
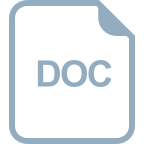
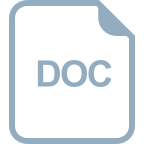
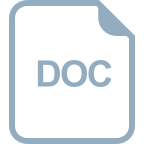
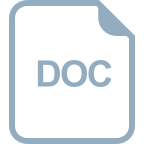
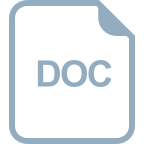
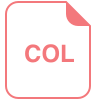