C++线性表的插入和删除
时间: 2023-11-07 07:05:53 浏览: 91
线性表的插入操作通常有两种情况:在指定位置插入元素和在末尾插入元素。删除操作也有两种情况:删除指定位置的元素和删除指定值的元素。下面我会分别介绍这四种操作的实现方法。
1. 在指定位置插入元素:
首先,需要判断插入位置是否合法,即判断插入位置是否大于等于0且小于等于线性表长度。若合法,则需要将插入位置后的元素依次向后移动一位,为新元素腾出位置,然后将新元素插入到指定位置上即可。
2. 在末尾插入元素:
直接将新元素添加到线性表的末尾即可。
3. 删除指定位置的元素:
首先,需要判断删除位置是否合法,即判断删除位置是否大于等于0且小于线性表长度。若合法,则将删除位置后的元素依次向前移动一位,覆盖掉待删除的元素,然后将线性表的长度减1即可。
4. 删除指定值的元素:
遍历整个线性表,找到第一个与指定值相等的元素所在的位置,然后按照删除指定位置的元素的方法进行处理。
请问还有其他
相关问题
线性表的插入和删除c++
线性表是一种数据结构,其中数据元素按照线性顺序排列,可以进行插入和删除操作。在C++中,可以使用数组或链表来实现线性表。下面是使用数组实现线性表插入和删除的示例代码:
```
#include <iostream>
using namespace std;
const int MAXSIZE = 100; // 线性表最大长度
// 定义线性表结构体
struct List {
int data[MAXSIZE]; // 数据元素
int length; // 线性表长度
};
// 初始化线性表
void InitList(List &L) {
L.length = 0;
}
// 插入数据元素
bool ListInsert(List &L, int pos, int elem) {
if (pos < 1 || pos > L.length + 1 || L.length >= MAXSIZE) {
return false; // 插入位置不合法或线性表已满
}
for (int i = L.length; i >= pos; i--) {
L.data[i] = L.data[i - 1]; // 数据元素后移
}
L.data[pos - 1] = elem; // 插入数据元素
L.length++; // 线性表长度加1
return true;
}
// 删除数据元素
bool ListDelete(List &L, int pos) {
if (pos < 1 || pos > L.length) {
return false; // 删除位置不合法
}
for (int i = pos; i < L.length; i++) {
L.data[i - 1] = L.data[i]; // 数据元素前移
}
L.length--; // 线性表长度减1
return true;
}
// 打印线性表
void PrintList(List L) {
for (int i = 0; i < L.length; i++) {
cout << L.data[i] << " ";
}
cout << endl;
}
int main() {
List L;
InitList(L);
ListInsert(L, 1, 1);
ListInsert(L, 2, 2);
ListInsert(L, 3, 3);
PrintList(L); // 输出:1 2 3
ListDelete(L, 2);
PrintList(L); // 输出:1 3
return 0;
}
```
使用链表实现线性表插入和删除的代码略有不同,具体实现可以参考其他资料。
c++线性表的数组实现
C++中可以使用数组来实现线性表。以下是一个简单的示例代码:
```cpp
#include <iostream>
using namespace std;
const int MAXSIZE = 100; // 线性表最大长度
class ArrayLinearList {
private:
int data[MAXSIZE]; // 存储线性表元素
int length; // 线性表长度
public:
ArrayLinearList() {
length = 0;
}
// 在位置i插入元素e
bool insert(int i, int e) {
if (i < 1 || i > length+1 || length == MAXSIZE) {
return false;
}
for (int j = length; j >= i; j--) {
data[j] = data[j-1];
}
data[i-1] = e;
length++;
return true;
}
// 删除位置i的元素
bool remove(int i) {
if (i < 1 || i > length) {
return false;
}
for (int j = i; j < length; j++) {
data[j-1] = data[j];
}
length--;
return true;
}
// 获取位置i的元素
bool get(int i, int& e) {
if (i < 1 || i > length) {
return false;
}
e = data[i-1];
return true;
}
// 输出线性表元素
void display() {
for (int i = 0; i < length; i++) {
cout << data[i] << " ";
}
cout << endl;
}
};
int main() {
ArrayLinearList list;
list.insert(1, 10);
list.insert(2, 20);
list.insert(3, 30);
list.display();
int e;
list.get(2, e);
cout << "位置2的元素为:" << e << endl;
list.remove(2);
list.display();
return 0;
}
```
以上代码实现了一个简单的数组线性表,包含了插入、删除、获取元素和输出元素等基本操作。
相关推荐
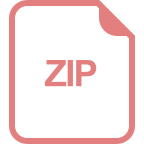












