c#如何统计datagridview中时间<datetime.now()的行数
时间: 2024-10-27 07:04:10 浏览: 14
在C#中,如果你想要统计DataGridView中所有当前日期(DateTime.Now())及之后的时间的行数,你可以按照以下步骤操作:
1. 首先,你需要遍历DataGridView的所有行。假设列名包含时间信息,例如"DateColumn",你可以获取该列的数据。
```csharp
DataGridView dataGridView = // 获取你的 DataGridView 对象
DateTime currentDate = DateTime.Now();
int count = 0;
foreach (DataGridViewRow row in dataGridView.Rows)
{
object dateCellValue = row.Cells["DateColumn"].Value; // 假设 "DateColumn" 是包含时间的列
if (dateCellValue != null && dateCellValue is DateTime && ((DateTime)dateCellValue).CompareTo(currentDate) >= 0)
{
count++;
}
}
```
2. 这段代码会检查每一行的指定单元格是否包含一个大于等于当前日期的时间值,如果是,则计数器加一。
相关问题
c# datagridview 单元格调用datetime
如果您想在 C# 的 DataGridView 中显示日期时间,可以将单元格的 ValueType 属性设置为 System.DateTime,然后将单元格的 Value 属性设置为 DateTime 类型的值。
以下是一个代码示例,用于设置 DataGridView 中的单元格为 DateTime 类型:
```csharp
// 设置 DataGridView 中的某个单元格为 DateTime 类型
DataGridViewCell cell = dataGridView1.Rows[0].Cells[0];
cell.ValueType = typeof(DateTime);
// 设置单元格的值为当前日期时间
cell.Value = DateTime.Now;
```
如果您需要将用户输入的日期时间保存到数据库中,可以使用 DateTime.Parse 方法将字符串转换为 DateTime 类型,然后将其存储到数据库中。例如:
```csharp
// 从 DataGridView 中获取用户输入的日期时间
string input = dataGridView1.Rows[0].Cells[0].Value.ToString();
DateTime dateTime = DateTime.Parse(input);
// 将日期时间保存到数据库中
// ...
```
希望这个回答能对您有所帮助。
C# DataGridView双缓冲实时计算时间
你可以通过以下方式实现C# DataGridView双缓冲实时计算时间:
1. 首先在你的程序中创建一个继承自DataGridView的子类,并重写OnPaint方法。
2. 在OnPaint方法中,创建一个Graphics对象并设置其SmoothingMode属性为AntiAlias。
3. 创建一个Bitmap对象,大小与DataGridView控件相同,然后创建一个Graphics对象,并将其绑定到Bitmap对象。
4. 在Bitmap对象上绘制DataGridView控件。
5. 在Bitmap对象上绘制当前时间,可以使用DateTime.Now.ToString()方法获取当前时间的字符串表示。
6. 将Bitmap对象绘制到控件的Graphics对象上。
7. 最后调用base.OnPaint(e)方法绘制其它元素。
以下是示例代码:
```csharp
public class DoubleBufferedDataGridView : DataGridView
{
protected override void OnPaint(PaintEventArgs e)
{
base.OnPaint(e);
using (var graphics = CreateGraphics())
{
graphics.SmoothingMode = SmoothingMode.AntiAlias;
var bitmap = new Bitmap(ClientSize.Width, ClientSize.Height);
using (var bitmapGraphics = Graphics.FromImage(bitmap))
{
// 绘制DataGridView控件
base.OnPaint(new PaintEventArgs(bitmapGraphics, e.ClipRectangle));
// 绘制当前时间
var timeString = DateTime.Now.ToString();
var timeFont = new Font("Arial", 12);
var timeBrush = new SolidBrush(Color.Black);
var timeLocation = new PointF(ClientSize.Width - 100, ClientSize.Height - 20);
bitmapGraphics.DrawString(timeString, timeFont, timeBrush, timeLocation);
}
// 将Bitmap对象绘制到控件的Graphics对象上
graphics.DrawImage(bitmap, e.ClipRectangle, e.ClipRectangle, GraphicsUnit.Pixel);
}
}
}
```
你可以将这个子类的实例化对象替换掉原来的DataGridView控件,这样就可以实现双缓冲和实时计算时间了。
阅读全文
相关推荐
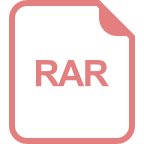
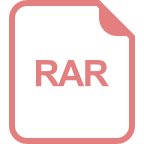
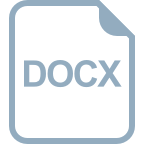













