用C语言编程求一个二元关系的传递闭包
时间: 2024-12-28 16:30:41 浏览: 12
在C语言中,求一个二元关系的传递闭包通常涉及到图论的概念,尤其是当你处理有向图或依赖关系时。一个简单的实现可以通过邻接矩阵或邻接表存储图,然后通过深度优先搜索(DFS)或广度优先搜索(BFS)来找到所有从给定节点可达的节点集合。
以下是一个简化的示例,使用邻接列表来表示二元关系,并使用BFS算法:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
typedef struct List {
Node* head;
} List;
// 添加边到图
void addEdge(List* graph, int src, int dest) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = dest;
newNode->next = graph->head;
graph->head = newNode;
}
// 检查是否在闭包中
bool inClosure(List* closure, int node) {
Node* current = closure->head;
while (current != NULL) {
if (current->data == node)
return true;
current = current->next;
}
return false;
}
// 添加到达节点到闭包
void addToClosure(List* closure, int node) {
Node** tail = &closure->head;
for(Node* current = closure->head; current != NULL; current = current->next) {
if(current->data == node)
break;
tail = ¤t->next;
}
*tail = (Node*)malloc(sizeof(Node));
(*tail)->data = node;
(*tail)->next = NULL;
}
// 寻找传递闭包
List findTransitiveClosure(List* graph, int startNode) {
List closure;
closure.head = NULL;
bool visited[startNode + 1];
// 初始化并标记开始节点
memset(visited, false, sizeof(bool) * (startNode + 1));
visited[startNode] = true;
// 使用BFS
Node* queue = (Node*)malloc(sizeof(Node));
queue->data = startNode;
queue->next = NULL;
closure.head = queue;
while (queue != NULL) {
int currentNode = queue->data;
queue = queue->next;
// 遍历当前节点的所有邻居
for (Node* neighbor = graph->head; neighbor != NULL; neighbor = neighbor->next) {
int neighborNode = neighbor->data;
if (!visited[neighborNode]) {
visited[neighborNode] = true;
addToClosure(&closure, neighborNode);
// 添加邻居到队列,继续搜索
queue = (Node*)malloc(sizeof(Node));
queue->data = neighborNode;
queue->next = NULL;
queue->next = closure.head;
closure.head = queue;
}
}
}
free(queue);
return closure;
}
int main() {
// 创建图并添加边...
List graph, result;
// ...
List* transitiveClosure = findTransitiveClosure(&graph, 0); // 求起始节点的传递闭包
// 输出闭包中的节点...
return 0;
}
```
在这个示例中,`findTransitiveClosure`函数实现了传递闭包的查找,而`main`函数则需要根据实际需求构造图并提供起始节点。注意这只是一个基础版本,实际应用中可能需要更复杂的数据结构和错误检查。
阅读全文
相关推荐













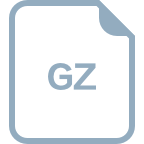
