在VHDL设计中,如何通过编程实现存储器的位扩展和字扩展?请提供对应的代码示例。
时间: 2024-11-16 21:26:19 浏览: 23
在VHDL中,存储器的位扩展和字扩展是通过修改存储器模块的信号和组件的连接方式来实现的。具体代码示例如下:
参考资源链接:[VHDL教程:存储器容量扩展技术解析](https://wenku.csdn.net/doc/1yzz5dmqpq?spm=1055.2569.3001.10343)
首先,我们定义一个存储器模块,该模块具有位扩展的能力。位扩展涉及到增加数据总线的宽度,而保持地址总线不变。代码如下:
```vhdl
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
use IEEE.NUMERIC_STD.ALL; -- 使用数值类型库
entity RAM_with_Bit_Extension is
Generic(
data_width : natural := 8; -- 数据位宽
address_width : natural := 10 -- 地址位宽
);
Port (
clk : in STD_LOGIC;
address : in STD_LOGIC_VECTOR(address_width-1 downto 0);
data_in : in STD_LOGIC_VECTOR(data_width*P-1 downto 0); -- P为位扩展倍数
data_out : out STD_LOGIC_VECTOR(data_width*P-1 downto 0);
we : in STD_LOGIC
);
end RAM_with_Bit_Extension;
architecture Behavioral of RAM_with_Bit_Extension is
-- 声明内部存储器数组
type RAM_Type is array (0 to 2**address_width-1) of STD_LOGIC_VECTOR(data_width-1 downto 0);
signal RAM : RAM_Type;
begin
process(clk)
begin
if rising_edge(clk) then
if we = '1' then
-- 写入操作,位扩展部分
for i in 0 to P-1 loop
RAM(to_integer(unsigned(address)))((i+1)*data_width-1 downto i*data_width) <= data_in((i+1)*data_width-1 downto i*data_width);
end loop;
end if;
end if;
end process;
-- 读取操作
data_out <= RAM(to_integer(unsigned(address)));
end Behavioral;
```
接下来,我们定义一个存储器模块,该模块具有字扩展的能力。字扩展涉及到增加地址总线的宽度,以支持更多的存储字。代码如下:
```vhdl
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
use IEEE.NUMERIC_STD.ALL;
entity RAM_with_Word_Extension is
Generic(
data_width : natural := 8;
address_width : natural := 10 -- 原始地址位宽
);
Port (
clk : in STD_LOGIC;
address : in STD_LOGIC_VECTOR(address_width+log2(P)-1 downto 0); -- P为字扩展倍数
data_in : in STD_LOGIC_VECTOR(data_width-1 downto 0);
data_out : out STD_LOGIC_VECTOR(data_width-1 downto 0);
we : in STD_LOGIC
);
end RAM_with_Word_Extension;
architecture Behavioral of RAM_with_Word_Extension is
-- 声明内部存储器数组
type RAM_Type is array (0 to 2**(address_width+log2(P))-1) of STD_LOGIC_VECTOR(data_width-1 downto 0);
signal RAM : RAM_Type;
begin
process(clk)
begin
if rising_edge(clk) then
if we = '1' then
RAM(to_integer(unsigned(address(address_width+log2(P)-1 downto log2(P))))) <= data_in;
end if;
end if;
end process;
-- 读取操作
data_out <= RAM(to_integer(unsigned(address(address_width+log2(P)-1 downto log2(P)))));
end Behavioral;
```
在位扩展的例子中,我们通过内部循环将数据分段写入到存储器中的相应位置。在字扩展的例子中,我们通过增加地址总线宽度并进行适当的地址解码,来实现对不同存储字的访问。以上代码示例展示了如何在VHDL中实现存储器的位扩展和字扩展,你可以根据具体需求调整数据位宽、地址位宽以及存储器大小等参数。
参考资源链接:[VHDL教程:存储器容量扩展技术解析](https://wenku.csdn.net/doc/1yzz5dmqpq?spm=1055.2569.3001.10343)
阅读全文
相关推荐
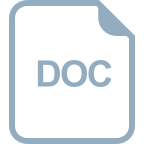
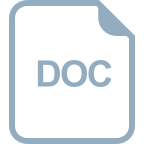
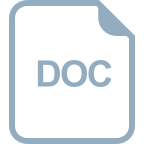

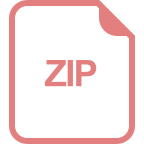
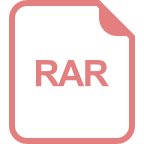
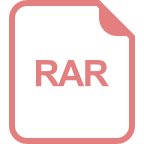
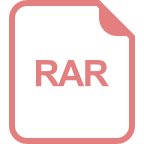
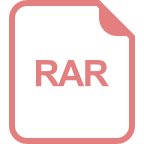
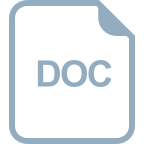
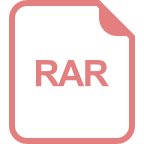
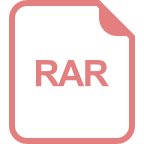
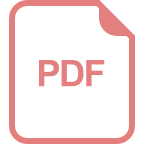
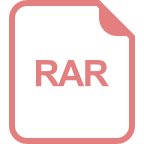
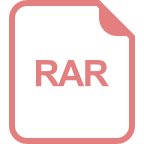
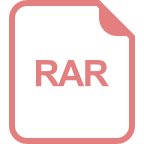
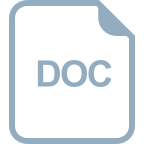
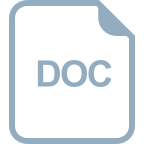