Linux的线程优先级反转示例
时间: 2024-12-25 10:17:40 浏览: 4
在Linux系统中,线程优先级反转是一个典型的现象,它发生在当一个高优先级的线程(通常由实时任务创建)尝试进入睡眠状态,而此时有一个低优先级的线程正在运行,并释放了CPU资源。在这种情况下,由于调度策略,低优先级线程可能会持续占用CPU,使得高优先级线程无法立即获得执行机会。
一个简单的示例如下:
```bash
#include <pthread.h>
#include <sched.h>
#define REALTIME_PRIORITY sched_get_priority_max(SCHED_FIFO)
#define USER_PRIORITY sched_get_priority_min(SCHED_NORMAL)
void *high_prio_task(void *arg) {
pthread_attr_t attr;
pthread_attr_init(&attr);
// 设置为实时优先级
pthread_attr_setinheritsched(&attr, PTHREAD_EXPLICIT_SCHED);
pthread_attr_setschedparam(&attr, &REALTIME_PRIORITY);
// 开始任务
while (1) {
sched_yield(); // 尝试让出CPU,等待调度
printf("High-priority task running.\n");
}
}
void *low_prio_task(void *arg) {
for (;;) {
printf("Low-priority task running.\n");
// 短暂睡眠模拟阻塞操作
sleep(1);
}
}
int main() {
pthread_t high_thread, low_thread;
// 创建并启动两个线程
pthread_create(&high_thread, NULL, high_prio_task, NULL);
pthread_create(&low_thread, NULL, low_prio_task, NULL);
// 等待线程结束
pthread_join(high_thread, NULL);
pthread_join(low_thread, NULL);
return 0;
}
```
在这个例子中,`high_prio_task`设置了实时优先级,试图通过`sched_yield()`主动让出控制权;而`low_prio_task`则是普通用户级任务。当`high_prio_task`试图休息时,`low_prio_task`会持续运行,造成线程优先级反转。
阅读全文
相关推荐
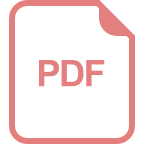
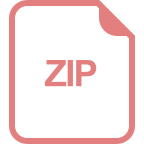
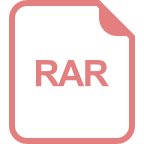
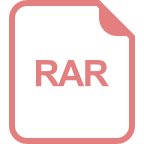
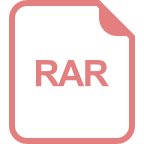
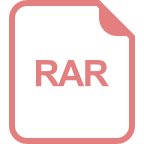
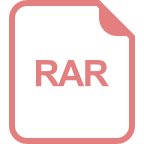
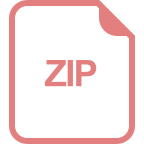
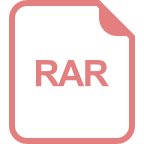
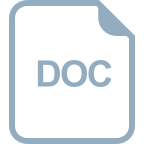
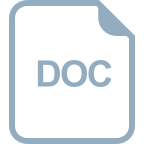
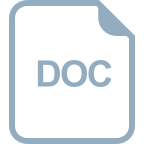
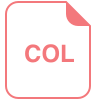
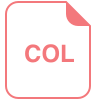
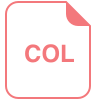



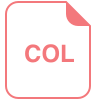
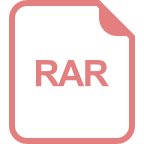