双线性卷积神经网络pytorch代码
时间: 2023-10-02 15:09:38 浏览: 75
双线性卷积神经网络是一种在处理图像分类和图像生成任务中非常有效的模型。下面是使用PyTorch实现双线性卷积神经网络的代码示例:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class BilinearCNN(nn.Module):
def __init__(self):
super(BilinearCNN, self).__init__()
self.conv1 = nn.Conv2d(3, 64, kernel_size=3, stride=1, padding=1)
self.conv2 = nn.Conv2d(64, 128, kernel_size=3, stride=1, padding=1)
self.fc1 = nn.Linear(128 * 32 * 32, 256) # Assuming input image size is 32x32
self.fc2 = nn.Linear(256, 10) # Assuming 10 classes for classification
def forward(self, x):
x = F.relu(self.conv1(x))
x = F.relu(self.conv2(x))
x = x.view(x.size(0), -1)
x = F.relu(self.fc1(x))
x = self.fc2(x)
return x
# 使用双线性卷积神经网络进行图像分类任务的例子
model = BilinearCNN()
input = torch.randn(1, 3, 32, 32) # assuming input image size is 32x32 with 3 channels
output = model(input)
```
相关推荐
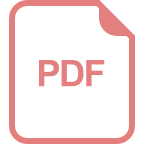














