补充上述所需其他代码
时间: 2024-10-13 17:02:45 浏览: 28
对于JSP页面的详细实现,这里给出一些关键部分的代码示例,假设你正在使用Spring MVC框架结合Thymeleaf模板引擎:
首先,在后端Controller中,可以处理表格数据的获取和操作:
```java
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
@Controller
public class TicketController {
private List<TicketItem> ticketItems; // 假设TicketItem是你的实体类
@GetMapping("/tickets")
public String showTickets(Model model) {
model.addAttribute("tickets", ticketItems); // 将数据传入模型
return "tickets"; // 返回Thymeleaf模板文件
}
@PostMapping("/add-ticket")
public String addTicket(TicketItem item) {
// 新增数据并更新列表
ticketItems.add(item);
// 通知视图更新
return "redirect:/tickets";
}
@PostMapping("/delete-ticket")
public String deleteTicket(Long id) {
// 根据id删除数据,更新列表
ticketItems.removeIf(ticket -> ticket.getId().equals(id));
// 通知视图更新
return "redirect:/tickets";
}
}
```
接下来,在Thymeleaf模板文件(如`tickets.html`)中,你可以嵌套循环展示表格:
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<!-- 引入CSS和JS -->
</head>
<body>
<table id="ticketList" th:object="${tickets}">
<thead>
<tr>
<th th:text="#{columnIndex}">序号</th>
<!-- 其他列 -->
</tr>
</thead>
<tbody>
<tr th:each="item : ${tickets}">
<td th:text="${item.index}">序号</td>
<!-- 根据item属性渲染其他列 -->
<td contenteditable="true" th:text="${item.preTaxAmount}">税前金额</td>
<!-- 类似地处理税额和总价 -->
</tr>
</tbody>
<tfoot>
<!-- 代码类似上面,动态计算总计 -->
</tfoot>
</table>
<div th:include="fragments/buttons :: button-section"></div>
</body>
</html>
```
在`fragments/buttons.html`中放置按钮区域:
```html
<section id="buttonSection">
<button id="addButton" th:text="#{addButton}" th:href="@{/add-ticket}"></button>
<button id="deleteButton" th:text="#{deleteButton}" th:href="@{/delete-ticket}"></button>
</section>
```
至于JavaScript部分,你可以参考之前提供的jQuery代码片段,将其放在`<script>`标签内或者外部.js文件中。注意,Thymeleaf本身支持简单的JavaScript操作,如`th:attr`、`th:onclick`等,具体是否需要使用jQuery取决于项目规模和个人喜好。
这只是一个基础的实现,实际代码可能需要根据项目需求和具体库进行调整。在开发过程中,记得测试每个功能,确保其正常工作。同时,考虑到安全性,不要直接在Thymeleaf模板中处理用户输入,而应通过HTTP请求传递到后端进行验证和处理。
阅读全文
相关推荐






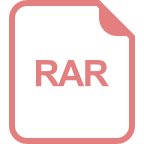












