CGAL实现闭合的轮廓线向内或者向外偏移
时间: 2024-09-20 10:09:17 浏览: 45
CGAL(Computational Geometry Algorithms Library),是一个开源的计算机辅助几何处理软件库,提供了一系列高级算法用于处理各种几何问题,包括二维和三维空间中的形状操作。对于闭合轮廓线的偏移,CGAL确实有相应的功能。
在CGAL中,你可以使用`Offset_2<Kernel>`或`Offset_3<Kernel>`模板来实现二维或三维轮廓线的向内或向外偏移。其中,`Kernel`是几何运算的核心,如坐标系统和几何构造函数。基本步骤通常如下:
1. 创建一个`Offset_2`或`Offset_3`对象,并传入合适的几何 Kernel 和初始轮廓。
2. 调用偏移函数,比如`offset()`,它接受偏移距离作为参数,可以是正值(向外偏移)或负值(向内偏移)。
3. 结果将是一个新的轮廓线,可能是包含原轮廓的一个圆环或壳体,具体取决于偏移方向。
例如,在二维情况下,可能会看到这样的代码片段:
```cpp
typedef CGAL::Exact_predicates_inexact_constructions_kernel Kernel;
typedef CGAL::Offset_2<Kernel> Offset_2;
// ...
Offset_2 offset(5); // 偏移量为5单位
std::vector<Point_2> new_contour = offset(outer_contour);
```
相关问题
CGAL实现封闭多边形缩放
CGAL (Computational Geometry Algorithms Library) 是一个开源的计算机辅助几何处理软件库,它提供了丰富的几何算法,包括对多边形的操作。要实现封闭多边形的缩放,你可以按照以下步骤在CGAL中操作:
1. **加载必要的模块**:首先,需要包含`CGAL::Polygon_2<Kernel>`模块,其中`Kernel`是一个几何运算的抽象层。
```cpp
#include <CGAL/Polygon_2.h>
#include <CGAL/Exact_predicates_inexact_constructions_kernel.h> // 使用精确几何
```
2. **创建多边形**:创建一个`Polygon_2`对象,并提供多边形顶点的坐标,表示初始大小的多边形。
```cpp
CGAL::Polygon_2<Kernel> polygon; // 假设顶点存储在一个向量中
std::vector<Point_2> vertices = ...; // 闭合路径的顶点集合
polygon = CGAL::Polygon_2<Kernel>(vertices.begin(), vertices.end());
```
3. **定义缩放因子**:确定一个缩放因子,比如0.5,这将把多边形缩小到原来的一半。
4. **应用缩放**:使用`scale()`函数,传入缩放因子以及多边形的一个点作为参照点(通常是原点 `(0, 0)`),对多边形进行缩放。
```cpp
double scale_factor = 0.5;
Point_2 origin(0, 0);
CGAL::scale(polygon, scale_factor, origin);
```
5. **验证结果**:最后检查缩放后的多边形是否按预期改变大小。
注意:这个过程假定你在使用CGAL的精确几何模式(`Exact_predicates_inexact_constructions_kernel`)。如果需要浮点精度,则可能会有所不同。
matlab中的scatteredInterpolant用CGAL实现
在 C++ 中,你可以使用 CGAL 库来实现 scatteredInterpolant 的功能。CGAL 提供了各种插值算法和数据结构,可以方便地实现散点数据插值。
以下是使用 CGAL 实现 scatteredInterpolant 的步骤:
1. 安装 CGAL 库:从官方网站下载 CGAL 库并安装到本地计算机。
2. 读取输入数据:从文件中读入散点数据,例如:x、y、z 坐标和对应的值。
3. 构建插值函数:使用 CGAL 库中的数据结构和算法构建插值函数。在这里,你可以使用 Delaunay 三角剖分或其他数据结构来存储散点数据。然后,使用插值算法(例如:Moving Least Squares、Natural Neighbor、Lagrange、Barycentric)来构建插值函数。
4. 进行插值计算:使用插值函数对输入数据进行插值计算。在 CGAL 库中,你可以使用函数对象或函数指针来执行这个功能。
5. 输出结果:将插值结果存储到文件中,例如:x、y、z 坐标和对应的值。
以下是一个使用 CGAL 库实现 scatteredInterpolant 的示例代码:
```c++
#include <iostream>
#include <fstream>
#include <CGAL/Exact_predicates_inexact_constructions_kernel.h>
#include <CGAL/Delaunay_triangulation_3.h>
#include <CGAL/interpolation_functions.h>
typedef CGAL::Exact_predicates_inexact_constructions_kernel K;
typedef CGAL::Delaunay_triangulation_3<K> Delaunay;
typedef Delaunay::Point Point;
typedef Delaunay::Vertex_handle Vertex_handle;
typedef Delaunay::Cell_handle Cell_handle;
typedef Delaunay::Facet Facet;
int main()
{
// 读取输入数据
std::ifstream ifs("input.txt");
std::vector<Point> points;
std::vector<double> values;
Point p;
double v;
while (ifs >> p >> v)
{
points.push_back(p);
values.push_back(v);
}
ifs.close();
// 构建插值函数
Delaunay dt(points.begin(), points.end());
auto interpolator = CGAL::Natural_neighbor_coordinates_3<Delaunay, double>(dt, values.begin());
// 进行插值计算
std::ofstream ofs("output.txt");
double x, y, z;
while (std::cin >> x >> y >> z)
{
double v = interpolator(Point(x, y, z));
ofs << x << " " << y << " " << z << " " << v << std::endl;
}
ofs.close();
return 0;
}
```
在这个示例代码中,我们使用 CGAL 库中的 Delaunay 三角剖分和 Natural Neighbor 插值算法来实现 scatteredInterpolant 的功能。在读入输入数据后,我们使用 Delaunay 三角剖分来存储散点数据,并使用 Natural Neighbor 插值算法来构建插值函数。然后,我们使用函数对象 `interpolator` 来进行插值计算,最后将插值结果存储到文件中。
阅读全文
相关推荐
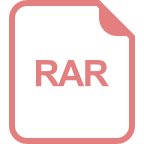
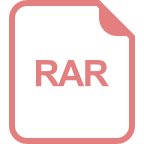
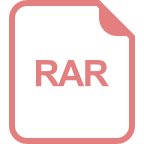
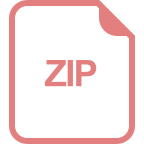
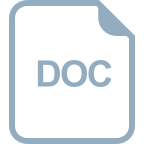









