python逻辑回归模型校准度
时间: 2023-11-30 17:43:11 浏览: 139
校准度是指模型预测结果与实际结果的一致性程度。在逻辑回归模型中,可以使用混淆矩阵和分类报告来评估模型的校准度。以下是一个简单的例子:
<<引用>>
```python
# 导入模型
from sklearn.linear_model import LogisticRegression
modelLR = LogisticRegression()
# 训练模型
modelLR.fit(X_train, y_train)
# 预测结果
y_pred = modelLR.predict(X_test)
# 评估模型
from sklearn.metrics import confusion_matrix, classification_report
print(confusion_matrix(y_test, y_pred))
print(classification_report(y_test, y_pred))
```
在上面的代码中,我们首先导入了逻辑回归模型,并使用训练数据拟合了模型。然后,我们使用测试数据进行了预测,并使用混淆矩阵和分类报告评估了模型的校准度。
混淆矩阵是一个2x2的矩阵,用于描述模型的预测结果和实际结果之间的关系。在混淆矩阵中,行表示实际结果,列表示预测结果。对角线上的元素表示正确预测的数量,非对角线上的元素表示错误预测的数量。
分类报告提供了更详细的评估结果,包括准确率、召回率、F1值等指标。准确率是指模型正确预测的样本占总样本数的比例,召回率是指模型正确预测的正样本占实际正样本数的比例,F1值是准确率和召回率的调和平均数。
相关问题
python画模型的校准曲线
在Python中,我们可以使用`sklearn`库中的`calibration_curve`函数来绘制模型的校准曲线。下面是一个简单的例子:
```python
from sklearn.datasets import make_classification
from sklearn.calibration import calibration_curve
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
import matplotlib.pyplot as plt
# 生成一个二分类数据集
X, y = make_classification(n_samples=1000, n_features=10, n_classes=2, random_state=42)
# 将数据集划分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 训练一个逻辑回归模型
clf = LogisticRegression(random_state=42)
clf.fit(X_train, y_train)
# 计算校准曲线的参数
probs = clf.predict_proba(X_test)[:, 1]
fop, mpv = calibration_curve(y_test, probs, n_bins=10)
# 绘制校准曲线
plt.plot([0, 1], [0, 1], linestyle='--')
plt.plot(mpv, fop, marker='.')
plt.show()
```
在上面的例子中,我们首先生成了一个二分类数据集,然后将数据集划分为训练集和测试集。接着,我们训练了一个逻辑回归模型,并使用`predict_proba`方法获取模型对测试集样本的预测概率。最后,我们使用`calibration_curve`函数计算校准曲线的参数,并使用`matplotlib`库绘制校准曲线。
python 校准曲线
校准曲线是一种用于评估模型校准效果的可视化工具。在Python中,我们可以使用sklearn库中的calibration_curve函数来绘制校准曲线。下面是一个简单的例子:
假设我们有一个二分类模型,我们可以使用以下代码来绘制校准曲线:
```python
from sklearn.calibration import calibration_curve
from sklearn.datasets import make_classification
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
import matplotlib.pyplot as plt
# 生成一个二分类数据集
X, y = make_classification(n_samples=10000, n_features=20, n_informative=10, n_redundant=0, random_state=42)
# 将数据集分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 训练一个逻辑回归模型
clf = LogisticRegression()
clf.fit(X_train, y_train)
# 绘制校准曲线
prob_pos = clf.predict_proba(X_test)[:, 1]
fraction_of_positives, mean_predicted_value = calibration_curve(y_test, prob_pos, n_bins=10)
plt.plot(mean_predicted_value, fraction_of_positives, "s-", label="Logistic Regression")
plt.plot([0, 1], [0, 1], "k--", label="Perfectly calibrated")
plt.legend()
plt.show()
```
在上面的代码中,我们首先生成了一个二分类数据集,然后将其分为训练集和测试集。接下来,我们训练了一个逻辑回归模型,并使用predict_proba函数获取测试集上的预测概率。最后,我们使用calibration_curve函数计算校准曲线的两个参数:fraction_of_positives和mean_predicted_value,并使用matplotlib库将其绘制出来。
阅读全文
相关推荐
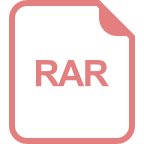
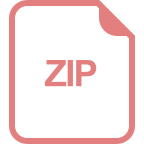


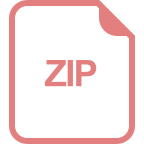
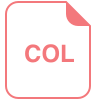
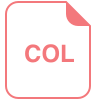
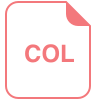
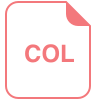
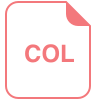
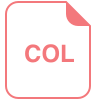
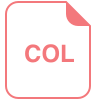
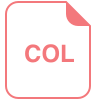
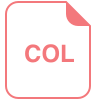
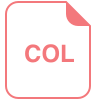