c语言编写一个固定换从的循环队列
时间: 2024-10-15 13:15:39 浏览: 26
在C语言中,固定大小的循环队列通常采用动态内存分配来处理。由于队列一旦初始化就不能改变大小,当队列满时需要进行溢出处理,而队列空时则需要有适当的空闲空间。下面是一个简单的固定大小循环队列的例子:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 10
typedef struct {
int* queue;
int head;
int tail;
int count;
} CircularQueue;
// 初始化循环队列
CircularQueue* createQueue() {
CircularQueue* cq = (CircularQueue*)malloc(sizeof(CircularQueue));
cq->queue = (int*)malloc(MAX_SIZE * sizeof(int));
cq->head = 0;
cq->tail = -1;
cq->count = 0;
return cq;
}
// 入队操作
void enqueue(CircularQueue* cq, int value) {
if (cq->count == MAX_SIZE) {
printf("Queue overflow.\n");
return;
}
if (cq->tail == -1) {
cq->tail = 0;
} else {
cq->tail = (cq->tail + 1) % MAX_SIZE;
}
cq->queue[cq->tail] = value;
cq->count++;
}
// 出队操作
int dequeue(CircularQueue* cq) {
if (cq->count == 0) {
printf("Queue underflow.\n");
return -1;
}
int value = cq->queue[(cq->head + 1) % MAX_SIZE];
if (cq->head == cq->tail) { // 队列空
cq->head = cq->tail = -1;
} else {
cq->head = (cq->head + 1) % MAX_SIZE;
}
cq->count--;
return value;
}
// 删除队列并释放内存
void destroyQueue(CircularQueue* cq) {
free(cq->queue);
free(cq);
}
// 示例用法
int main() {
CircularQueue* cq = createQueue();
enqueue(cq, 1);
enqueue(cq, 2);
enqueue(cq, 3);
printf("%d\n", dequeue(cq)); // 输出 1
printf("%d\n", dequeue(cq)); // 输出 2
destroyQueue(cq); // 清理资源
return 0;
}
阅读全文
相关推荐
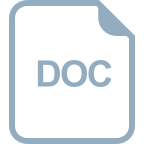
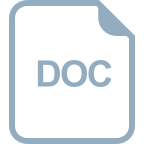
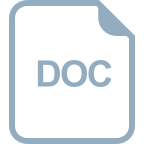
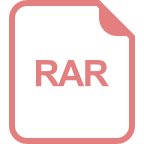

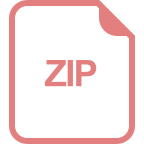
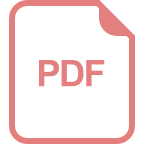
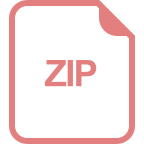
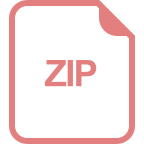
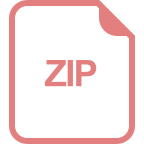
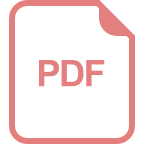
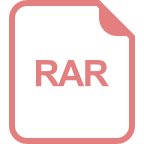
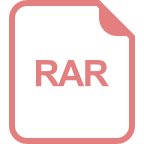
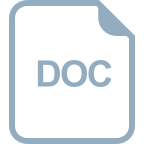
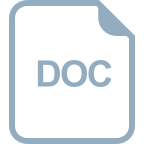
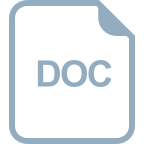
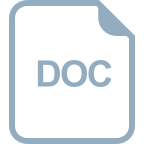
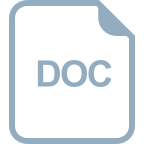