帮我写一份数据预处理代码 图像处理: 图像缩放和归一化:所有图像经过统一的缩放和像素值归一化处理,将像素值映射至[0, 1]区间,以提高模型训练的稳定性和收敛速度。 数据增强:训练集图像通过随机旋转、平移、缩放和水平翻转等增强技术,增加了数据的多样性和泛化能力。
时间: 2024-12-16 10:27:36 浏览: 20
当然可以。以下是一个简单的Python代码片段,使用PIL库进行图像预处理:
```python
import numpy as np
from PIL import Image
# 定义函数用于图像缩放和归一化
def preprocess_image(image_path):
# 打开图片
img = Image.open(image_path)
# 缩放图片到固定大小 (例如224x224),并转换为灰度图(对于某些模型)
img_resized = img.resize((224, 224))
if img.mode != 'L':
img_resized = img_resized.convert('L') # 转为灰度
# 将像素值归一化到[0, 1]
img_array = np.array(img_resized) / 255.0
return img_array.astype(np.float32)
# 定义数据增强函数
def data_augmentation(image, label):
# 随机增强操作,这里使用torchvision.transforms
transform = transforms.Compose([
transforms.RandomRotation(10),
transforms.RandomHorizontalFlip(),
transforms.RandomResizedCrop(224),
transforms.ToTensor(),
])
augmented_image = transform(image)
return augmented_image, label
# 使用示例
image_path = 'path_to_your_image.jpg'
preprocessed_img = preprocess_image(image_path)
augmented_data = data_augmentation(preprocessed_img, label)
```
阅读全文
相关推荐
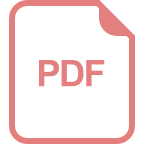
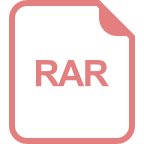
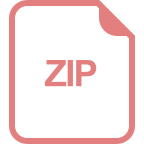















