第一步:采集人员佩戴安全帽和未佩戴安全帽图片作为数据集。对数据集进行分析和预处理,从而得到相关图片特征。第二步:选取合适的模型在训练集上进行训练,得到分类模型。第三步:最后在测试数据集上进行图片分类识别,获取选择模型相关性能指标。依次编写代码
时间: 2024-11-05 13:24:45 浏览: 4
好的,下面是按照您提供的步骤编写的代码示例:
### 第一步:数据集采集与预处理
#### 1.1 数据集采集
假设我们已经有一个文件夹 `dataset`,里面有两个子文件夹 `with_helmet` 和 `without_helmet`,分别存放佩戴安全帽和未佩戴安全帽的图片。
```python
import os
from PIL import Image
import numpy as np
import matplotlib.pyplot as plt
# 数据集路径
data_dir = 'dataset'
with_helmet_dir = os.path.join(data_dir, 'with_helmet')
without_helmet_dir = os.path.join(data_dir, 'without_helmet')
# 加载图片
def load_images_from_folder(folder):
images = []
for filename in os.listdir(folder):
img_path = os.path.join(folder, filename)
if img_path.endswith(".jpg") or img_path.endswith(".png"):
img = Image.open(img_path).convert('RGB')
img = img.resize((64, 64)) # 统一图片大小
images.append(np.array(img))
return np.array(images)
with_helmet_images = load_images_from_folder(with_helmet_dir)
without_helmet_images = load_images_from_folder(without_helmet_dir)
# 合并数据集
X = np.concatenate((with_helmet_images, without_helmet_images), axis=0)
y = np.array([1] * len(with_helmet_images) + [0] * len(without_helmet_images))
# 打乱数据集
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
#### 1.2 数据预处理
归一化图片数据,并将其转换为浮点数。
```python
# 归一化
X_train = X_train.astype('float32') / 255.0
X_test = X_test.astype('float32') / 255.0
```
### 第二步:模型选择与训练
#### 2.1 构建卷积神经网络 (CNN) 模型
使用 Keras 构建一个简单的 CNN 模型。
```python
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense, Dropout
model = Sequential([
Conv2D(32, (3, 3), activation='relu', input_shape=(64, 64, 3)),
MaxPooling2D((2, 2)),
Conv2D(64, (3, 3), activation='relu'),
MaxPooling2D((2, 2)),
Conv2D(128, (3, 3), activation='relu'),
MaxPooling2D((2, 2)),
Flatten(),
Dense(128, activation='relu'),
Dropout(0.5),
Dense(1, activation='sigmoid')
])
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
```
#### 2.2 训练模型
```python
history = model.fit(X_train, y_train, epochs=20, batch_size=32, validation_data=(X_test, y_test))
```
### 第三步:模型评估
#### 3.1 在测试集上评估模型性能
```python
loss, accuracy = model.evaluate(X_test, y_test)
print(f'Test Loss: {loss:.4f}')
print(f'Test Accuracy: {accuracy:.4f}')
```
#### 3.2 可视化训练过程
```python
plt.figure(figsize=(12, 4))
plt.subplot(1, 2, 1)
plt.plot(history.history['accuracy'], label='Training Accuracy')
plt.plot(history.history['val_accuracy'], label='Validation Accuracy')
plt.legend()
plt.title('Accuracy Over Epochs')
plt.subplot(1, 2, 2)
plt.plot(history.history['loss'], label='Training Loss')
plt.plot(history.history['val_loss'], label='Validation Loss')
plt.legend()
plt.title('Loss Over Epochs')
plt.show()
```
以上代码涵盖了从数据采集、预处理、模型构建、训练到评估的完整流程。希望这对您有所帮助!如果有任何问题或需要进一步的帮助,请随时告诉我。
阅读全文
相关推荐
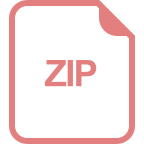
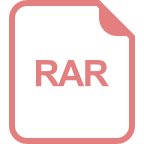
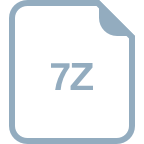

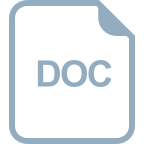

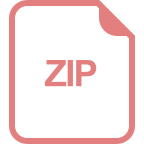
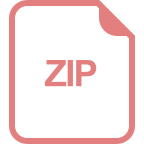
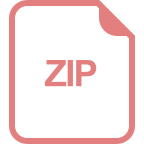
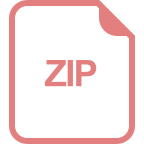
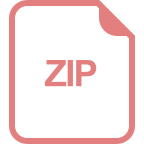
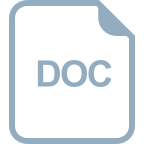
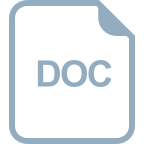
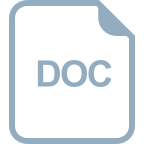
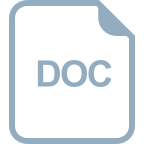
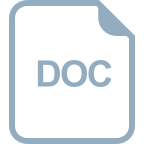
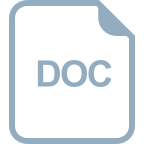
