将attn从(8,3168,200)映射到(8,200)的pytorch代码
时间: 2024-10-22 11:25:06 浏览: 10
在PyTorch中,如果你有一个注意力机制(Attention)层,其输出形状为`(batch_size, sequence_length, hidden_dim)`,例如 `(8, 3168, 200)`,并且你想将其压缩到`(batch_size, hidden_dim)`,通常是为了得到每个位置的全局上下文向量,你可以使用线性变换(Linear或者nn.Linear模块)或者简单的池化操作(如平均池或最大池)。这里是一个简单的例子:
```python
import torch
# 假设attn是你的输入张量
attn = torch.randn(8, 3168, 200) # (batch_size, sequence_length, hidden_dim)
# 定义一个线性层(全连接层),将最后一维降维
projection_layer = nn.Linear(200, 200)
compressed_attn = projection_layer(attn.mean(dim=1)) # 使用平均池来压缩,也可以用 `attn.max(dim=1)[0]` 替换
print(compressed_attn.shape) # 输出:(8, 200)
```
这个代码首先计算了attn在sequence维度上的平均值,然后通过线性层将其转换成 `(8, 200)` 的形状。
相关问题
cross-attention代码pytorch
以下是一个简单的 PyTorch 实现交叉注意力(cross-attention)的代码:
```python
import torch
import torch.nn as nn
class CrossAttention(nn.Module):
def __init__(self, input_dim):
super(CrossAttention, self).__init__()
self.input_dim = input_dim
self.linear_q = nn.Linear(input_dim, input_dim)
self.linear_k = nn.Linear(input_dim, input_dim)
self.linear_v = nn.Linear(input_dim, input_dim)
def forward(self, x1, x2):
q = self.linear_q(x1)
k = self.linear_k(x2)
v = self.linear_v(x2)
attn_weights = torch.matmul(q, k.transpose(1, 2))
attn_weights = torch.softmax(attn_weights, dim=-1)
output = torch.matmul(attn_weights, v)
return output
```
其中,`input_dim` 表示输入的特征维度,`x1` 和 `x2` 分别表示两个输入张量。在 `forward` 方法中,我们首先通过三个线性层将输入张量映射到相同的特征空间,然后计算注意力权重并将其应用于第二个输入张量 `x2` 的值上,最后返回加权和。
帮我用pytorch写出SparseAttention代码
以下是使用PyTorch实现的Sparse Attention代码:
```python
import torch
class SparseAttention(torch.nn.Module):
def __init__(self, dim_in, dim_out, n_heads, dropout=0.0):
super(SparseAttention, self).__init__()
self.dim_in = dim_in
self.dim_out = dim_out
self.n_heads = n_heads
self.dropout = dropout
self.Q = torch.nn.Linear(dim_in, dim_out * n_heads)
self.K = torch.nn.Linear(dim_in, dim_out * n_heads)
self.V = torch.nn.Linear(dim_in, dim_out * n_heads)
self.dropout_layer = torch.nn.Dropout(dropout)
self.out = torch.nn.Linear(dim_out * n_heads, dim_in)
def forward(self, x):
batch_size, seq_len, _ = x.size()
Q = self.Q(x).view(batch_size, seq_len, self.n_heads, self.dim_out)
K = self.K(x).view(batch_size, seq_len, self.n_heads, self.dim_out)
V = self.V(x).view(batch_size, seq_len, self.n_heads, self.dim_out)
attn = (Q @ K.transpose(-2, -1)) / (self.dim_out ** 0.5)
attn = torch.nn.functional.softmax(attn, dim=-1)
attn = self.dropout_layer(attn)
x = (attn @ V).transpose(1, 2).contiguous().view(batch_size, seq_len, -1)
x = self.out(x)
return x
```
这个代码实现了一个带有dropout的稀疏自注意力层,可以用于NLP中的文本分类、序列标注等任务。在这个实现中,我们使用了线性层来进行Q、K、V的变换,并使用了PyTorch的softmax函数来计算注意力权重。注意力权重和V的乘积采用了batched matrix multiplication的方式进行计算,以提高计算效率。最后,我们使用了一个线性层来将注意力计算的结果映射回原始维度。
阅读全文
相关推荐
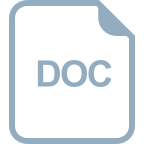
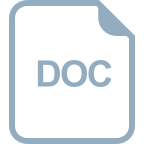
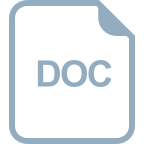
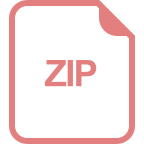
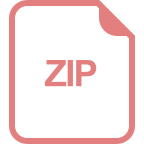
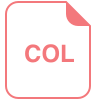
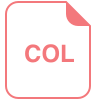
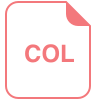
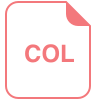
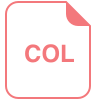







