如何编写python来计算从公元1年1月1日起至用户输入特定公元年月日(包括闰年的情况)的总天数、小时数、分钟数和秒数?
时间: 2024-11-14 14:22:37 浏览: 4
为了计算从公元1年1月1日至用户输入的特定公元年月日的总天数,我们可以分步操作:
1. **确定日期与公元1年1月1日的相对位置**[^2]:
使用`datetime`模块,先定义一个基线日期`base_date`(如`date(1, 1, 1)`),然后创建用户输入的日期`target_date`。
```python
from datetime import date
base_date = date(1, 1, 1)
target_date = date(year, month, day) # 假设year, month, day分别为用户输入的年、月、日
```
2. **计算天数差**:
计算两个日期之间的差异,得到`timedelta`对象,可以从中提取总天数。
```python
delta = target_date - base_date
total_days = delta.days
```
3. **额外计算时间部分**:
如果需要计算小时数、分钟数和秒数,还需要考虑闰年的额外天数(如果有的话)。你可以借助`is_leap_year`函数来判断是否为闰年。
```python
leap_days = sum(is_leap_year(year) for year in range(1, target_date.year + 1))
total_hours = total_days * 24
total_minutes = total_hours * 60
total_seconds = total_minutes * 60
```
完整代码示例:
```python
from datetime import date, timedelta
def is_leap_year(year):
if (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0):
return True
else:
return False
# 用户输入
year, month, day = int(input("请输入年份: ")), int(input("请输入月份: ")), int(input("请输入日期: "))
base_date = date(1, 1, 1)
target_date = date(year, month, day)
delta = target_date - base_date
total_days = delta.days
leap_days = sum(is_leap_year(year) for year in range(1, target_date.year + 1))
total_hours = total_days * 24
total_minutes = total_hours * 60
total_seconds = total_minutes * 60
print(f"总天数: {total_days}")
print(f"总小时数: {total_hours}")
print(f"总分钟数: {total_minutes}")
print(f"总秒数: {total_seconds}")
```
阅读全文
相关推荐
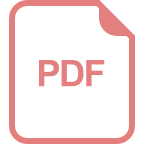
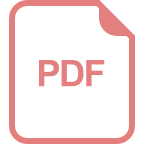
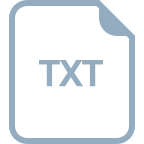


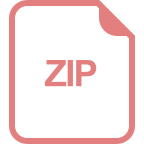
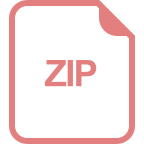






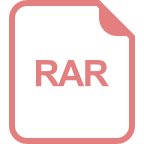