ts中instanceof
时间: 2024-09-28 11:11:56 浏览: 37
在TypeScript (TS) 中,`instanceof` 关键字用于检查一个值是否属于某个特定的类或者它的超类。它是一个运算符,通常用于动态类型检查,特别是在需要对对象进行类型确认的时候。
例如:
```typescript
class Animal {
speak() {
console.log('Animal speaks');
}
}
class Dog extends Animal {
speak() {
console.log('Dog barks');
}
}
let dogInstance = new Dog();
if (dogInstance instanceof Dog) {
console.log('This is a Dog instance');
} else if (dogInstance instanceof Animal) {
console.log('This is an Animal instance, but not a Dog specifically');
}
```
在这个例子中,`dogInstance instanceof Dog` 返回 `true`,因为 `dogInstance` 是 `Dog` 类的一个实例。而 `dogInstance instanceof Animal` 也返回 `true`,因为所有 `Dog` 都是 `Animal` 的子类。
相关问题
ts 如何判断instanceof interface
在 TypeScript 中,我们可以使用 `instanceof` 运算符来检查一个对象是否为某个类的实例。对于接口(interface)而言,它们是在编译时进行类型检查的,并不存在运行时的实例化对象,因此无法使用 `instanceof` 运算符来判断一个对象是否为某个接口的实例。
如果你需要在 TypeScript 中判断一个对象是否实现了某个接口,可以使用类型断言(Type Assertion)来实现。例如,假设有一个接口名为 `MyInterface`,你可以使用以下代码判断一个对象 `obj` 是否实现了该接口:
```typescript
interface MyInterface {
// 接口定义
}
const obj: any = { /* 对象定义 */ };
if ((obj as MyInterface).myMethod) {
// obj 实现了 MyInterface 接口
} else {
// obj 未实现 MyInterface 接口
}
```
在上述代码中,我们使用了类型断言 `(obj as MyInterface)` 将 `obj` 转换为 `MyInterface` 接口类型,并检查该对象是否实现了接口中定义的方法或属性。需要注意的是,这种方式并不能完全保证对象是否实现了接口,因为类型断言并不进行类型检查,仅仅是告诉编译器在后续代码中将该对象视为某种类型。因此,在使用类型断言判断对象是否实现接口时,需要保证该对象确实实现了接口中定义的所有方法或属性。
ts中怎么获取某个对象的类
在TypeScript (TS) 中,你可以通过`instanceof`操作符来检查一个对象是否属于特定的类。如果你有一个变量引用了一个对象实例,你可以这样做:
```typescript
let obj: YourClass = new YourClass(); // 创建一个YourClass类型的对象
// 检查obj是否是YourClass的实例
if (obj instanceof YourClass) {
console.log('obj is an instance of YourClass');
}
```
如果你想获取对象的实际类名(即它的构造函数),可以使用`Object.getPrototypeOf()` 或 `Reflect.getPrototypeOf()`方法,结合`.constructor`属性:
```typescript
const className = Reflect.getPrototypeOf(obj).constructor.name;
console.log(className); // 输出 'YourClass'
```
这里假设`YourClass`是你想检查的具体类。
阅读全文
相关推荐
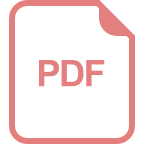
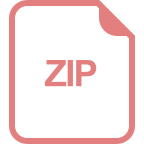
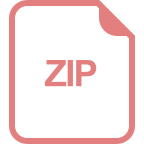
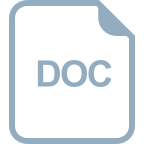












