python 文本 向量
时间: 2025-01-07 07:14:24 浏览: 4
### Python 中的文本向量化方法和库
#### doc2vec 方法
通过 `gensim` 库中的 Doc2Vec 实现文档级别的向量化。训练完成后,可以使用训练好的模型来获取文本的向量表示。对于一个给定的文本,可以通过调用 `infer_vector()` 函数并传入分词后的文本列表来获得其向量表示[^1]。
```python
from gensim.models.doc2vec import Doc2Vec, TaggedDocument
from nltk.tokenize import word_tokenize
model = Doc2Vec.load("path_to_model") # 加载预训练模型
vector = model.infer_vector(word_tokenize("我喜欢使用Python"))
print(vector)
```
#### CountVectorizer 类实现词频特征提取
在 scikit-learn 的 `feature_extraction.text` 模块中提供了 `CountVectorizer` 类用于提取词频特征,并利用词袋模型将文本转换成数值型特征矩阵。该过程会统计每篇文档中各个词语出现次数作为特征值[^2]。
```python
from sklearn.feature_extraction.text import CountVectorizer
corpus = [
"这是一条关于中国的新闻",
"美国经济正在复苏"
]
vectorizer = CountVectorizer()
X = vectorizer.fit_transform(corpus)
print(X.toarray())
print(vectorizer.get_feature_names_out())
```
#### TF-IDF 向量化技术
TfidfVectorizer 是基于TF-IDF (Term Frequency-Inverse Document Frequency) 权重计算方式的一种文本向量化工具。它不仅考虑了单词频率还加入了逆文档频率因子以减少常见词汇的影响权重[^3]。
```python
from sklearn.feature_extraction.text import TfidfVectorizer
tfidf = TfidfVectorizer()
response = tfidf.fit_transform(corpus).toarray()
print(response)
print(tfidf.vocabulary_)
```
#### Word2Vec 训练自定义语料库
Word2Vec 可以用来构建大规模文本数据集上的分布式表示形式。下面展示了如何读取文件并通过 Gensim 工具包创建自己的 Word2Vec 模型[^4]:
```python
import logging
from gensim.models import Word2Vec
from gensim.models.word2vec import LineSentence
logging.basicConfig(format='%(asctime)s : %(levelname)s : %(message)s', level=logging.INFO)
def train_word2vec(input_file_path, output_model_path):
with open(input_file_path, 'r') as f:
sentences = LineSentence(f)
model = Word2Vec(sentences=sentences,
size=192,
window=5,
min_count=5,
workers=9)
model.save(output_model_path)
if __name__ == "__main__":
input_file = "./data/reduce_zhiwiki.txt"
output_model = "zhiwiki_news.word2vec"
train_word2vec(input_file, output_model)
```
阅读全文
相关推荐





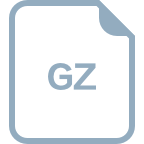











